用c语言写代码 1. 实现单循环链表的初始化、求数据元素个数、插入、删除、取数据元素等操作; 2. 用插入法建立带头结点的单循环链表;
时间: 2024-10-08 19:11:12 浏览: 40
在C语言中,我们可以创建一个简单的单循环链表结构以及相关的操作函数。这里是一个基本的示例:
首先,我们定义链表节点的数据结构:
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
```
1. 初始化链表:
```c
Node* createList() {
Node* head = (Node*)malloc(sizeof(Node)); // 创建头节点
head->data = 0; // 初始化值(可以自定义)
head->next = head; // 设置头节点的next指针指向自身形成循环
return head;
}
```
2. 求数据元素个数(这里假设链表是非空的):
```c
int getLength(Node* head) {
int count = 1; // 初始化计数器
Node* temp = head->next;
while (temp != head) {
count++;
temp = temp->next;
}
return count;
}
```
3. 插入节点:
```c
void insertNode(Node** head, int value, int position) {
if (position == 1) { // 插入到头节点
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = *head;
*head = newNode;
} else {
Node* current = *head;
for (int i = 1; i < position - 1 && current->next != head; i++) {
current = current->next;
}
if (current->next == head) {
printf("Invalid position!\n");
return;
}
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = current->next;
current->next = newNode;
}
}
```
4. 删除节点:
```c
void deleteNode(Node** head, int value) {
Node* temp = *head;
Node* prev = NULL;
while (temp != NULL && temp->data != value) {
prev = temp;
temp = temp->next;
}
if (temp == NULL) {
printf("Value not found in the list.\n");
return;
}
if (prev == NULL) { // 删除头节点
*head = temp->next;
} else {
prev->next = temp->next;
}
free(temp);
}
```
5. 取数据元素:
```c
int getData(Node* head, int position) {
Node* current = head;
for (int i = 1; i <= position; i++) {
if (current->next == head) {
printf("Index out of range!\n");
return -1;
}
current = current->next;
}
return current->data;
}
```
阅读全文
相关推荐
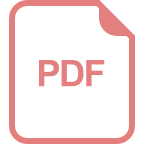
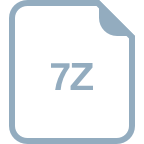
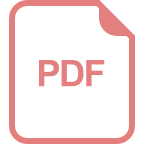









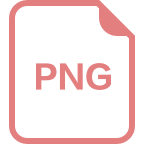
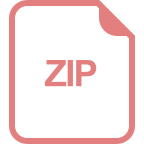
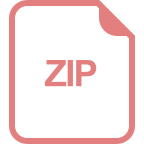
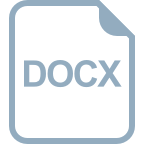