pthread_create使用示例
时间: 2024-12-13 11:21:39 浏览: 2
`pthread_create`是POSIX线程库提供的函数,用于创建新的线程。下面是一个简单的C语言示例,展示了如何使用它:
```c
#include <stdio.h>
#include <pthread.h>
// 定义线程执行的函数
void* thread_function(void* arg) {
int thread_num = (int)arg; // 线程参数
printf("Thread %d started.\n", thread_num);
sleep(2); // 模拟一些工作时间
printf("Thread %d finished.\n", thread_num);
return NULL;
}
int main() {
pthread_t threads[5]; // 创建的线程数组
int i;
// 对每个线程创建并启动
for (i = 0; i < 5; ++i) {
if (pthread_create(&threads[i], NULL, &thread_function, (void*)i)) { // 创建新线程
perror("Error creating thread");
exit(1);
}
}
// 等待所有线程完成
for (i = 0; i < 5; ++i) {
if (pthread_join(threads[i], NULL)) { // 等待线程结束
perror("Error joining thread");
exit(1);
}
}
printf("All threads completed.\n");
return 0;
}
```
在这个例子中,`main`函数首先定义了一个线程函数`thread_function`,然后在`for`循环中使用`pthread_create`创建了五个线程,每个线程传递一个不同的整数作为参数,并执行`thread_function`。`pthread_join`用于等待所有线程执行完毕。
阅读全文
相关推荐
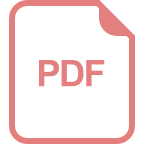
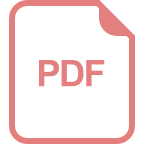
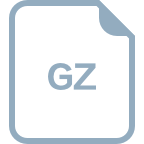











