在Linux系统中,如何使用pthread库安全地创建线程,并控制线程的退出以及资源的回收?请结合pthread_create、pthread_exit和pthread_join函数给出示例代码。
时间: 2024-10-31 16:26:23 浏览: 47
为了安全有效地使用pthread库在Linux环境中进行线程的创建、管理和退出,理解相关函数的工作机制是至关重要的。这里推荐您参考《Linux用户空间线程编程详解:pthread库与关键操作》,它将提供全面的理论知识和实际操作指导。
参考资源链接:[Linux用户空间线程编程详解:pthread库与关键操作](https://wenku.csdn.net/doc/5om5u4mc75?spm=1055.2569.3001.10343)
在Linux中使用pthread库创建线程时,通常会使用pthread_create函数。这个函数需要指定线程的属性、线程要执行的函数以及该函数的参数。示例代码如下:
```c
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
// 线程函数定义
void* thread_function(void* arg) {
// 执行任务...
printf(
参考资源链接:[Linux用户空间线程编程详解:pthread库与关键操作](https://wenku.csdn.net/doc/5om5u4mc75?spm=1055.2569.3001.10343)
相关问题
如何在Linux环境下使用pthread_create创建多个线程,并使用pthread_join等待所有线程完成?
在Linux环境下,利用pthread_create创建多个线程并在之后使用pthread_join等待所有线程完成,是多线程编程中常见的需求。首先,为了创建多个线程,可以使用一个循环结构来重复调用pthread_create函数。创建的每个线程都应保存其对应的线程标识符pthread_t,以便后续管理。完成后,主线程应调用pthread_join等待每个子线程完成执行。为了等待多个线程,主线程可以循环调用pthread_join,直到所有线程都被成功join。示例代码如下(省略具体线程任务代码,此处略):在这个过程中,需要确保线程函数正确返回,以便在pthread_join时获取线程的返回值。如果线程函数中未定义返回值,则pthread_join的第二个参数可以为NULL。创建多个线程时,还需注意线程安全问题,比如使用互斥锁来保护共享资源,避免数据竞争和不一致。建议深入阅读《Linux下使用pthread_create创建线程详解》,这本教程不仅详细介绍了创建和等待线程的方法,还涉及了多线程编程中的其他重要概念,如线程属性设置、线程同步机制等,是解决当前问题并进一步提升Linux下多线程编程能力的宝贵资源。
参考资源链接:[Linux下使用pthread_create创建线程详解](https://wenku.csdn.net/doc/6412b53bbe7fbd1778d426b1?spm=1055.2569.3001.10343)
如何在Linux环境下利用pthread库创建、管理和退出线程?请提供创建线程、退出线程和回收资源的具体示例。
在Linux环境下进行多线程编程时,pthread库是一个强大的工具,它允许开发者创建、同步和管理线程。要正确地使用pthread库来控制线程的生命周期,包括创建、管理和退出线程,你需要熟悉几个核心函数:pthread_create(), pthread_exit(), 和 pthread_join()。以下是如何使用这些函数的具体示例:
参考资源链接:[Linux用户空间线程编程详解:pthread库与关键操作](https://wenku.csdn.net/doc/5om5u4mc75?spm=1055.2569.3001.10343)
1. 创建线程:
pthread_create()函数用于创建新线程,你需要提供一个pthread_t类型的变量来接收新创建的线程标识符。函数的原型如下:
int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine) (void *), void *arg);
例如,创建一个线程并传递参数:
```c
#include <pthread.h>
#include <stdio.h>
void* thread_function(void* arg) {
// 线程函数内容
printf(
参考资源链接:[Linux用户空间线程编程详解:pthread库与关键操作](https://wenku.csdn.net/doc/5om5u4mc75?spm=1055.2569.3001.10343)
阅读全文
相关推荐
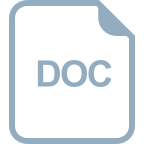
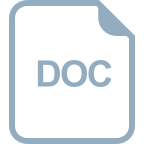
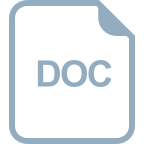



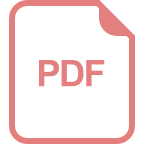







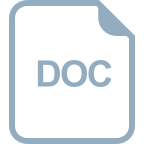
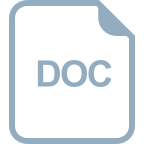
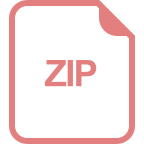