pthread_create 创建线程并使用全局变量
时间: 2024-04-27 22:17:50 浏览: 26
pthread_create是一个函数,用于创建一个新的线程。它的原型如下:
```c
int pthread_create(pthread_t *thread, const pthread_attr_t *attr,
void *(*start_routine) (void *), void *arg);
```
其中,`thread`是一个指向pthread_t类型的指针,用于存储新线程的标识符;`attr`是一个指向pthread_attr_t类型的指针,用于设置线程的属性;`start_routine`是一个指向函数的指针,该函数将作为新线程的入口点;`arg`是传递给`start_routine`函数的参数。
在创建线程后,可以使用全局变量来在主线程和新线程之间进行通信。全局变量是在所有线程中都可见的变量,因此可以在不同的线程中读取和修改它们的值。
下面是一个示例代码,演示了如何使用pthread_create创建线程并使用全局变量:
```c
#include <stdio.h>
#include <pthread.h>
int global_var = 0; // 全局变量
void* thread_func(void* arg) {
global_var = 10; // 在新线程中修改全局变量的值
printf("New thread: global_var = %d\n", global_var);
pthread_exit(NULL);
}
int main() {
pthread_t thread;
pthread_create(&thread, NULL, thread_func, NULL); // 创建新线程
global_var = 5; // 在主线程中修改全局变量的值
printf("Main thread: global_var = %d\n", global_var);
pthread_join(thread, NULL); // 等待新线程结束
return 0;
}
```
在上面的示例中,我们创建了一个全局变量`global_var`,然后在主线程和新线程中分别修改它的值。在主线程中,我们将`global_var`设置为5,在新线程中,我们将其设置为10。最后,我们使用`pthread_join`函数等待新线程结束。
相关推荐
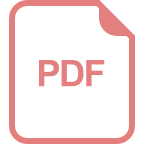
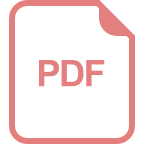
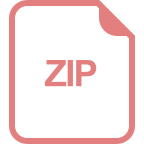















