请使用pthread库完成如下功能,2个线程更新一个全局变量count
时间: 2024-10-16 08:21:14 浏览: 37
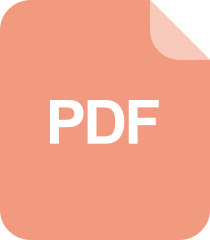
浅析Linux下一个简单的多线程互斥锁的例子
在使用C语言的POSIX线程库(pthread)来实现两个线程同时更新全局变量count时,你需要遵循一些基本步骤:
1. **包含头文件**:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
```
2. **初始化全局变量**:
```c
pthread_mutex_t count_mutex; // 互斥锁用于同步对count的访问
int count = 0; // 全局计数器
```
3. **创建互斥锁**:
```c
pthread_mutex_init(&count_mutex, NULL);
```
4. **定义共享数据结构和线程函数**:
```c
void* increment_count(void* arg) {
pthread_mutex_lock(&count_mutex); // 加锁
count++;
printf("Thread %d incremented count to %d\n", pthread_self(), count);
pthread_mutex_unlock(&count_mutex); // 解锁
return NULL;
}
```
5. **创建并启动线程**:
```c
int thread_id[2];
for (int i = 0; i < 2; ++i) {
pthread_create(&thread_id[i], NULL, increment_count, NULL);
}
```
6. **等待所有线程结束**:
```c
for (int i = 0; i < 2; ++i) {
pthread_join(thread_id[i], NULL);
}
```
7. **清理资源**:
```c
pthread_mutex_destroy(&count_mutex);
```
在这个例子中,`increment_count` 函数被两个线程并发地调用,通过互斥锁保证了对全局变量`count`的原子性更新。
阅读全文
相关推荐
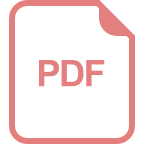
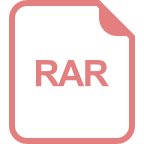


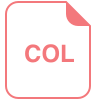












