C语言)有两个线程,共享一个全局变量count,count的初始值为0。这两个线程的任务是:线程1负责将count的的数值加到10,而线程2而负责在线程1将count加到10之后将count输出后清零,这交替循环。 截图上传运行结果和代码
时间: 2024-11-29 11:18:36 浏览: 22
在C语言中,实现这种线程同步和数据共享的情况通常需要使用互斥锁(mutex)或条件变量(condition variable)来避免竞态条件。这里是一个简单的示例代码片段,展示了如何使用`pthread`库来实现这个需求:
```c
#include <stdio.h>
#include <pthread.h>
// 全局变量
int count = 0;
pthread_mutex_t mutex; // 互斥锁
pthread_cond_t cond; // 条件变量
void *thread1(void *arg) {
while (true) {
pthread_mutex_lock(&mutex);
if (count >= 10) {
printf("Thread 1: Count is %d, exiting...\n", count);
pthread_mutex_unlock(&mutex);
break;
} else {
count++;
printf("Thread 1: Incrementing count to %d\n", count);
pthread_cond_signal(&cond); // 唤醒等待的线程
}
pthread_mutex_unlock(&mutex);
}
return NULL;
}
void *thread2(void *arg) {
while (true) {
pthread_mutex_lock(&mutex);
while (count != 10) { // 等待count达到10
pthread_cond_wait(&cond, &mutex);
}
printf("Thread 2: Outputting count as %d and resetting to 0\n", count);
count = 0;
pthread_mutex_unlock(&mutex);
sleep(1); // 暂停一下再开始下一轮
}
return NULL;
}
int main() {
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&cond, NULL);
pthread_t thread1_id, thread2_id;
pthread_create(&thread1_id, NULL, thread1, NULL);
pthread_create(&thread2_id, NULL, thread2, NULL);
pthread_join(thread1_id, NULL);
pthread_join(thread2_id, NULL);
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&cond);
return 0;
}
```
在这个例子中,`thread1` 加1并唤醒`thread2`,然后`thread2` 输出并清零count。`mutex` 确保了同时只有一个线程访问count,而`cond` 则用于线程间的通信。
运行这个程序,你应该能看到交替打印出线程的操作过程。请注意,为了演示效果,实际应用中应考虑更多的错误处理和退出条件。
阅读全文
相关推荐
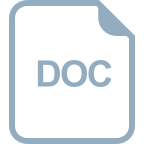
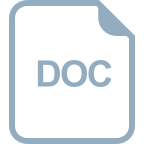
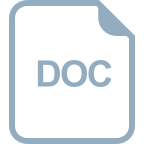

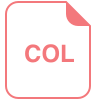
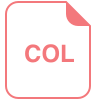
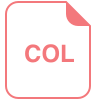
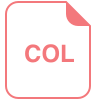
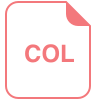
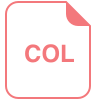
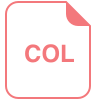
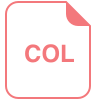
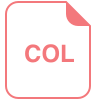
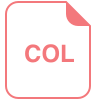
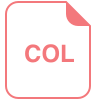
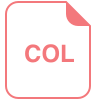
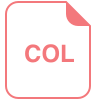
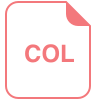