. 请使用pthread库完成如下功能,2个线程更新一个全局变量count, 每个线程增加 count 的值若干次,采取互斥更新方式,保证总计数正 确。
时间: 2024-10-16 21:21:19 浏览: 27
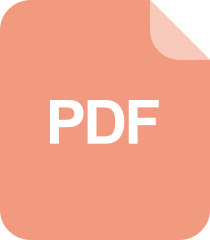
浅析Linux下一个简单的多线程互斥锁的例子
在使用C语言的POSIX线程库(pthread)实现这个功能时,你需要创建两个线程,每个线程都需要访问并更新全局变量`count`。由于需要互斥地修改这个变量以避免数据竞争,你可以利用`pthread_mutex_t`结构来实现临界区。
以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <pthread.h>
// 全局变量,表示计数
int count = 0;
// 互斥锁
pthread_mutex_t lock;
void *thread_function(void *arg) {
int num_updates = *((int *)arg); // 获取传入的线程次数
for (int i = 0; i < num_updates; ++i) {
pthread_mutex_lock(&lock); // 加锁
count++; // 更新计数
printf("Thread %d incremented count to %d\n", pthread_self(), count);
pthread_mutex_unlock(&lock); // 解锁
}
return NULL;
}
int main() {
pthread_t thread1, thread2;
int num_updates_thread1 = 5;
int num_updates_thread2 = 7;
// 初始化互斥锁
pthread_mutex_init(&lock, NULL);
// 创建线程并传入更新次数
if (pthread_create(&thread1, NULL, thread_function, &num_updates_thread1)) {
perror("Error creating thread 1");
return -1;
}
if (pthread_create(&thread2, NULL, thread_function, &num_updates_thread2)) {
perror("Error creating thread 2");
return -1;
}
// 等待线程结束
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
// 锁定之后关闭互斥锁
pthread_mutex_destroy(&lock);
printf("Final count: %d\n", count);
return 0;
}
```
在这个例子中,`thread_function`函数会在线程内部递增`count`并保持互斥,通过`pthread_mutex_lock()`获取锁,`pthread_mutex_unlock()`释放锁。`main`函数负责创建线程、传递更新次数,并在所有操作完成后销毁互斥锁。
阅读全文
相关推荐
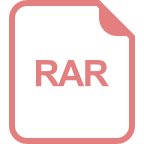
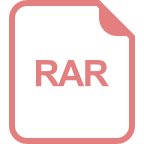




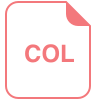










