java获取framebuffer
时间: 2023-11-01 22:45:58 浏览: 149
在Java中获取framebuffer可以使用Java OpenGL (JOGL)库。以下是获取framebuffer的步骤:
1. 导入JOGL库并创建一个GLAutoDrawable对象。
2. 在初始化方法中设置OpenGL上下文并创建framebuffer对象。
3. 在绘制方法中使用OpenGL渲染场景并将渲染结果绑定到framebuffer对象。
4. 使用OpenGL读取framebuffer对象的像素数据。
下面是获取framebuffer的示例代码:
import com.jogamp.opengl.*;
import com.jogamp.opengl.util.*;
import java.nio.*;
public class FramebufferExample implements GLEventListener {
private GLAutoDrawable drawable;
private int framebufferId;
private int textureId;
private int width;
private int height;
public static void main(String[] args) {
GLProfile profile = GLProfile.getDefault();
GLCapabilities capabilities = new GLCapabilities(profile);
GLCanvas canvas = new GLCanvas(capabilities);
FramebufferExample example = new FramebufferExample();
canvas.addGLEventListener(example);
JFrame frame = new JFrame("Framebuffer Example");
frame.getContentPane().add(canvas);
frame.setSize(800, 600);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
@Override
public void init(GLAutoDrawable drawable) {
this.drawable = drawable;
GL2 gl = drawable.getGL().getGL2();
// Create framebuffer object
int[] fbIds = new int[1];
gl.glGenFramebuffers(1, fbIds, 0);
framebufferId = fbIds[0];
// Create texture object
int[] texIds = new int[1];
gl.glGenTextures(1, texIds, 0);
textureId = texIds[0];
// Bind texture to framebuffer
gl.glBindFramebuffer(GL2.GL_FRAMEBUFFER, framebufferId);
gl.glBindTexture(GL2.GL_TEXTURE_2D, textureId);
gl.glTexImage2D(GL2.GL_TEXTURE_2D, 0, GL2.GL_RGB, 800, 600, 0, GL2.GL_RGB, GL2.GL_UNSIGNED_BYTE, null);
gl.glFramebufferTexture2D(GL2.GL_FRAMEBUFFER, GL2.GL_COLOR_ATTACHMENT0, GL2.GL_TEXTURE_2D, textureId, 0);
// Check framebuffer status
int status = gl.glCheckFramebufferStatus(GL2.GL_FRAMEBUFFER);
if (status != GL2.GL_FRAMEBUFFER_COMPLETE) {
throw new RuntimeException("Framebuffer is not complete: " + status);
}
// Unbind framebuffer
gl.glBindFramebuffer(GL2.GL_FRAMEBUFFER, 0);
}
@Override
public void display(GLAutoDrawable drawable) {
GL2 gl = drawable.getGL().getGL2();
// Bind framebuffer
gl.glBindFramebuffer(GL2.GL_FRAMEBUFFER, framebufferId);
// Render scene to framebuffer
gl.glClearColor(0.0f, 0.0f, 1.0f, 1.0f);
gl.glClear(GL2.GL_COLOR_BUFFER_BIT);
// Unbind framebuffer
gl.glBindFramebuffer(GL2.GL_FRAMEBUFFER, 0);
// Read framebuffer data
ByteBuffer buffer = ByteBuffer.allocateDirect(width * height * 4);
gl.glBindTexture(GL2.GL_TEXTURE_2D, textureId);
gl.glGetTexImage(GL2.GL_TEXTURE_2D, 0, GL2.GL_RGBA, GL2.GL_UNSIGNED_BYTE, buffer);
buffer.rewind();
byte[] pixels = new byte[width * height * 4];
buffer.get(pixels);
// Process framebuffer data
// ...
}
@Override
public void reshape(GLAutoDrawable drawable, int x, int y, int width, int height) {
this.width = width;
this.height = height;
}
@Override
public void dispose(GLAutoDrawable drawable) {
GL2 gl = drawable.getGL().getGL2();
// Delete framebuffer and texture objects
gl.glDeleteFramebuffers(1, new int[] {framebufferId}, 0);
gl.glDeleteTextures(1, new int[] {textureId}, 0);
}
}
在上面的示例代码中,我们在init方法中创建了一个framebuffer对象和一个texture对象,并将它们绑定在一起。在display方法中,我们先绑定framebuffer对象,然后使用OpenGL渲染场景,并将渲染结果绑定到texture对象上。最后,我们使用glGetTexImage方法读取texture对象的像素数据,并进行处理。在reshape方法中,我们记录了窗口的宽度和高度。在dispose方法中,我们删除了framebuffer和texture对象。
阅读全文
相关推荐





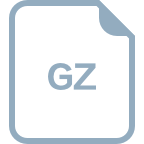












