用stm32f103写一段MFRCC522的驱动代码
时间: 2024-03-15 09:46:10 浏览: 13
好的,下面是一个简单的MFRC522驱动代码,适用于STM32F103系列芯片。
首先需要定义MFRC522芯片的一些寄存器地址和命令:
```c
#define MFRC522_REG_COMMAND 0x01 // Command register
#define MFRC522_REG_FIFO 0x09 // FIFO buffer
#define MFRC522_REG_STATUS 0x0A // Status register
#define MFRC522_REG_MODE 0x11 // Control register
#define MFRC522_CMD_IDLE 0x00 // No action, cancels current command execution
#define MFRC522_CMD_AUTHENT 0x0E // Authentication key
#define MFRC522_CMD_RECEIVE 0x08 // Receive data
#define MFRC522_CMD_TRANSMIT 0x04 // Transmit data
#define MFRC522_CMD_TRANSCEIVE 0x0C // Transmit and receive data
#define MFRC522_MODE_MIFARE 0x00 // Mifare standard authentication
```
接下来定义一些函数来操作MFRC522芯片:
```c
void MFRC522_Reset(void)
{
GPIO_ResetBits(GPIOA, GPIO_Pin_3); // Reset the MFRC522 by setting the Reset pin low
Delay_Ms(50); // Wait for the MFRC522 to complete the reset process
GPIO_SetBits(GPIOA, GPIO_Pin_3); // Release the Reset pin
}
uint8_t MFRC522_Read(uint8_t addr)
{
uint8_t data;
SPI_CS_LOW(); // Select the MFRC522 by setting the CS pin low
SPI_SendByte(addr << 1 | 0x80); // Send the address with the read bit set
data = SPI_ReceiveByte(); // Receive the data
SPI_CS_HIGH(); // Deselect the MFRC522 by setting the CS pin high
return data;
}
void MFRC522_Write(uint8_t addr, uint8_t data)
{
SPI_CS_LOW(); // Select the MFRC522 by setting the CS pin low
SPI_SendByte(addr << 1 & 0x7E); // Send the address with the write bit cleared
SPI_SendByte(data); // Send the data
SPI_CS_HIGH(); // Deselect the MFRC522 by setting the CS pin high
}
void MFRC522_AntennaOn(void)
{
uint8_t value = MFRC522_Read(MFRC522_REG_MODE);
if ((value & 0x03) != 0x03) {
MFRC522_Write(MFRC522_REG_MODE, value | 0x03);
}
}
void MFRC522_AntennaOff(void)
{
MFRC522_Write(MFRC522_REG_MODE, MFRC522_Read(MFRC522_REG_MODE) & ~0x03);
}
void MFRC522_Init(void)
{
MFRC522_Reset(); // Reset the MFRC522
MFRC522_Write(MFRC522_REG_COMMAND, MFRC522_CMD_IDLE); // Stop any currently executing command
MFRC522_Write(MFRC522_REG_MODE, MFRC522_MODE_MIFARE); // Set the MFRC522 to Mifare mode
MFRC522_AntennaOn(); // Turn on the antenna
}
```
最后在主函数中调用初始化函数和其他需要的函数即可:
```c
int main(void)
{
SPI_Config(); // Configure the SPI interface
MFRC522_Init(); // Initialize the MFRC522
while (1) {
// Your code here
}
}
```
以上代码只是一个简单的示例,具体实现还需要根据实际需求进行调整和完善。
相关推荐
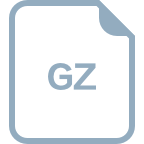















