c++实现打开一个50g的二进制文件,修改前四个字节,后面不变,给出示例
时间: 2024-01-03 22:05:34 浏览: 152
下面是一个使用C++实现打开二进制文件并修改前四个字节的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <vector>
int main()
{
// 打开二进制文件
std::ifstream file("filename.bin", std::ios::binary | std::ios::in);
if (!file.is_open()) {
std::cerr << "Failed to open file" << std::endl;
return -1;
}
// 读取前四个字节
std::vector<char> data(4);
file.read(data.data(), 4);
if (file.gcount() != 4) {
std::cerr << "Failed to read file" << std::endl;
return -1;
}
// 修改前四个字节
data[0] = 0x12;
data[1] = 0x34;
data[2] = 0x56;
data[3] = 0x78;
// 关闭文件
file.close();
// 打开二进制文件以写入模式
std::ofstream outfile("filename.bin", std::ios::binary | std::ios::out);
if (!outfile.is_open()) {
std::cerr << "Failed to open file" << std::endl;
return -1;
}
// 写入修改后的前四个字节
outfile.write(data.data(), 4);
if (!outfile) {
std::cerr << "Failed to write file" << std::endl;
return -1;
}
// 关闭文件
outfile.close();
return 0;
}
```
在这个示例代码中,我们使用了C++标准库的`ifstream`和`ofstream`类来打开和操作二进制文件。首先我们使用`ifstream`打开文件以读取模式,然后使用`read()`方法读取前四个字节。接着,我们修改这个字节数组的前四个字节,并且关闭文件。然后我们使用`ofstream`打开文件以写入模式,使用`write()`方法将修改后的字节数组写入文件中,最后关闭文件。
阅读全文
相关推荐
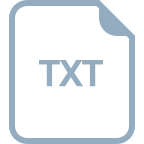



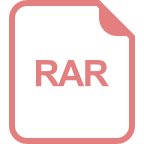
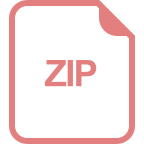
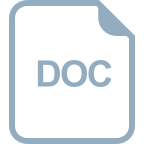
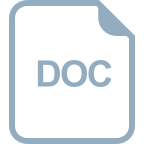
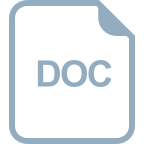
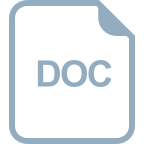
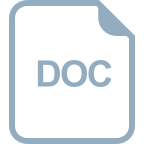




