首页python TypeError:the JSON object must be str,bytes or bytearray,not dict
python TypeError:the JSON object must be str,bytes or bytearray,not dict
时间: 2024-05-10 21:15:35 浏览: 24
这个错误通常是因为你尝试将一个字典对象转换为 JSON 字符串时,传递给了一个需要字符串、字节或字节数组的函数。解决这个问题的方法是将字典对象转换为 JSON 字符串。
你可以使用 Python 内置的 `json` 模块来将字典对象转换为 JSON 字符串。具体的做法是,使用 `json.dumps()` 方法将字典转换为 JSON 字符串,然后将字符串传递给需要的函数。
下面是一个例子:
```python
import json
my_dict = {"name": "John", "age": 30}
# 将字典对象转换为 JSON 字符串
json_str = json.dumps(my_dict)
# 将 JSON 字符串传递给需要的函数
my_function(json_str)
```
在这个例子中,`my_dict` 是一个字典对象,我们使用 `json.dumps()` 方法将其转换为 JSON 字符串,然后将字符串传递给函数 `my_function()`。
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
最新推荐
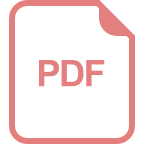
解决Python 异常TypeError: cannot concatenate 'str' and 'int' objects
主要介绍了解决Python 异常TypeError: cannot concatenate 'str' and 'int' objects,具有很好的参考价值,希望对大家有所帮助。一起跟随小编过来看看吧
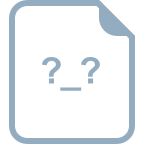
SecondactivityMainActivity.java
SecondactivityMainActivity.java
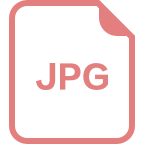
mmexport1719207093976.jpg
mmexport1719207093976.jpg
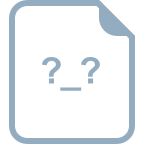
百度贴吧 安装包 全球最大的中文社区互动平台
百度贴吧安装包的相关信息如下:
应用介绍:
百度贴吧APP是全球最大中文社区互动平台,多样化的互动模式及板块都可自由进入,与吧友们一起互动交流。
它是一个以兴趣主题聚合志同道合者的互动平台,用户可以在这里畅所欲言,聊游戏、聊数码、聊动漫、聊收藏、聊手艺、聊运动等,满足各类用户的兴趣需求。
功能特点:
好内容,超懂你:提供专属内容推荐,根据用户兴趣推送相关贴吧和话题。
找同好,聊兴趣:用户可以轻松找到志同道合的吧友,进行深入的交流和讨论。
追热点,玩热梗:快速获取前沿热梗,与吧友一起分享讨论。
找游戏,看榜单:首页游戏中心提供丰富游戏资源和榜单,满足游戏玩家的需求。
主要功能:
兴趣频道:分类展现,精彩内容沉浸体验更过瘾。
话题热榜:热点榜单一手掌握,方便用户了解最新动态。
吧友评价:真实评价一目了然,帮助用户了解贴吧和吧友的情况。
贴吧好物:商品橱窗,吧友推荐一键购买更便捷,为用户提供购物便利。
更新日志:
百度贴吧APP不断更新优化,解决已知问题,提升用户体验。例如,增加了会员装扮升级、小尾巴、头像框等个性化设置,新增了虚拟形象、吧友互助等有趣玩法。
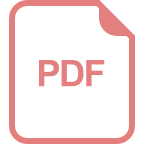
2024年东南亚3-甲氧基丙胺(MOPA)市场深度研究及预测报告.pdf
东南亚位于我国倡导推进的“一带一路”海陆交汇地带,作为当今全球发展最为迅速的地区之一,近年来区域内生产总值实现了显著且稳定的增长。根据东盟主要经济体公布的最新数据,印度尼西亚2023年国内生产总值(GDP)增长5.05%;越南2023年经济增长5.05%;马来西亚2023年经济增速为3.7%;泰国2023年经济增长1.9%;新加坡2023年经济增长1.1%;柬埔寨2023年经济增速预计为5.6%。
东盟国家在“一带一路”沿线国家中的总体GDP经济规模、贸易总额与国外直接投资均为最大,因此有着举足轻重的地位和作用。当前,东盟与中国已互相成为双方最大的交易伙伴。中国-东盟贸易总额已从2013年的443亿元增长至 2023年合计超逾6.4万亿元,占中国外贸总值的15.4%。在过去20余年中,东盟国家不断在全球多变的格局里面临挑战并寻求机遇。2023东盟国家主要经济体受到国内消费、国外投资、货币政策、旅游业复苏、和大宗商品出口价企稳等方面的提振,经济显现出稳步增长态势和强韧性的潜能。
本调研报告旨在深度挖掘东南亚市场的增长潜力与发展机会,分析东南亚市场竞争态势、销售模式、客户偏好、整体市场营商环境,为国内企业出海开展业务提供客观参考意见。
本文核心内容:
市场空间:全球行业市场空间、东南亚市场发展空间。
竞争态势:全球份额,东南亚市场企业份额。
销售模式:东南亚市场销售模式、本地代理商
客户情况:东南亚本地客户及偏好分析
营商环境:东南亚营商环境分析
本文纳入的企业包括国外及印尼本土企业,以及相关上下游企业等,部分名单
QYResearch是全球知名的大型咨询公司,行业涵盖各高科技行业产业链细分市场,横跨如半导体产业链(半导体设备及零部件、半导体材料、集成电路、制造、封测、分立器件、传感器、光电器件)、光伏产业链(设备、硅料/硅片、电池片、组件、辅料支架、逆变器、电站终端)、新能源汽车产业链(动力电池及材料、电驱电控、汽车半导体/电子、整车、充电桩)、通信产业链(通信系统设备、终端设备、电子元器件、射频前端、光模块、4G/5G/6G、宽带、IoT、数字经济、AI)、先进材料产业链(金属材料、高分子材料、陶瓷材料、纳米材料等)、机械制造产业链(数控机床、工程机械、电气机械、3C自动化、工业机器人、激光、工控、无人机)、食品药品、医疗器械、农业等。邮箱:market@qyresearch.com
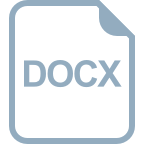
BSC绩效考核指标汇总 (2).docx
BSC(Balanced Scorecard,平衡计分卡)是一种战略绩效管理系统,它将企业的绩效评估从传统的财务维度扩展到非财务领域,以提供更全面、深入的业绩衡量。在提供的文档中,BSC绩效考核指标主要分为两大类:财务类和客户类。
1. 财务类指标:
- 部门费用的实际与预算比较:如项目研究开发费用、课题费用、招聘费用、培训费用和新产品研发费用,均通过实际支出与计划预算的百分比来衡量,这反映了部门在成本控制上的效率。
- 经营利润指标:如承保利润、赔付率和理赔统计,这些涉及保险公司的核心盈利能力和风险管理水平。
- 人力成本和保费收益:如人力成本与计划的比例,以及标准保费、附加佣金、续期推动费用等与预算的对比,评估业务运营和盈利能力。
- 财务效率:包括管理费用、销售费用和投资回报率,如净投资收益率、销售目标达成率等,反映公司的财务健康状况和经营效率。
2. 客户类指标:
- 客户满意度:通过包装水平客户满意度调研,了解产品和服务的质量和客户体验。
- 市场表现:通过市场销售月报和市场份额,衡量公司在市场中的竞争地位和销售业绩。
- 服务指标:如新契约标保完成度、续保率和出租率,体现客户服务质量和客户忠诚度。
- 品牌和市场知名度:通过问卷调查、公众媒体反馈和总公司级评价来评估品牌影响力和市场认知度。
BSC绩效考核指标旨在确保企业的战略目标与财务和非财务目标的平衡,通过量化这些关键指标,帮助管理层做出决策,优化资源配置,并驱动组织的整体业绩提升。同时,这份指标汇总文档强调了财务稳健性和客户满意度的重要性,体现了现代企业对多维度绩效管理的重视。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
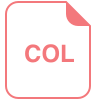
【进阶】Flask中的会话与用户管理

# 2.1 用户注册和登录
### 2.1.1 用户注册表单的设计和验证
用户注册表单是用户创建帐户的第一步,因此至关重要。它应该简单易用,同时收集必要的用户信息。
* **字段设计:**表单应包含必要的字段,如用户名、电子邮件和密码。
* **验证:**表单应验证字段的格式和有效性,例如电子邮件地址的格式和密码的强度。
* **错误处理:**表单应优雅地处理验证错误,并提供清晰的错误消

卷积神经网络实现手势识别程序
卷积神经网络(Convolutional Neural Network, CNN)在手势识别中是一种非常有效的机器学习模型。CNN特别适用于处理图像数据,因为它能够自动提取和学习局部特征,这对于像手势这样的空间模式识别非常重要。以下是使用CNN实现手势识别的基本步骤:
1. **输入数据准备**:首先,你需要收集或获取一组带有标签的手势图像,作为训练和测试数据集。
2. **数据预处理**:对图像进行标准化、裁剪、大小调整等操作,以便于网络输入。
3. **卷积层(Convolutional Layer)**:这是CNN的核心部分,通过一系列可学习的滤波器(卷积核)对输入图像进行卷积,以
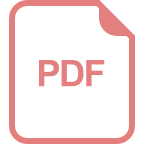
BSC资料.pdf
"BSC资料.pdf"
战略地图是一种战略管理工具,它帮助企业将战略目标可视化,确保所有部门和员工的工作都与公司的整体战略方向保持一致。战略地图的核心内容包括四个相互关联的视角:财务、客户、内部流程和学习与成长。
1. **财务视角**:这是战略地图的最终目标,通常表现为股东价值的提升。例如,股东期望五年后的销售收入达到五亿元,而目前只有一亿元,那么四亿元的差距就是企业的总体目标。
2. **客户视角**:为了实现财务目标,需要明确客户价值主张。企业可以通过提供最低总成本、产品创新、全面解决方案或系统锁定等方式吸引和保留客户,以实现销售额的增长。
3. **内部流程视角**:确定关键流程以支持客户价值主张和财务目标的实现。主要流程可能包括运营管理、客户管理、创新和社会责任等,每个流程都需要有明确的短期、中期和长期目标。
4. **学习与成长视角**:评估和提升企业的人力资本、信息资本和组织资本,确保这些无形资产能够支持内部流程的优化和战略目标的达成。
绘制战略地图的六个步骤:
1. **确定股东价值差距**:识别与股东期望之间的差距。
2. **调整客户价值主张**:分析客户并调整策略以满足他们的需求。
3. **设定价值提升时间表**:规划各阶段的目标以逐步缩小差距。
4. **确定战略主题**:识别关键内部流程并设定目标。
5. **提升战略准备度**:评估并提升无形资产的战略准备度。
6. **制定行动方案**:根据战略地图制定具体行动计划,分配资源和预算。
战略地图的有效性主要取决于两个要素:
1. **KPI的数量及分布比例**:一个有效的战略地图通常包含20个左右的指标,且在四个视角之间有均衡的分布,如财务20%,客户20%,内部流程40%。
2. **KPI的性质比例**:指标应涵盖财务、客户、内部流程和学习与成长等各个方面,以全面反映组织的绩效。
战略地图不仅帮助管理层清晰传达战略意图,也使员工能更好地理解自己的工作如何对公司整体目标产生贡献,从而提高执行力和组织协同性。