使用json.loads(result)中提示raise TypeError(f'the JSON object must be str, bytes or bytearray, ' TypeError: the JSON object must be str, bytes or bytearray, not dict
时间: 2024-04-14 16:28:29 浏览: 24
`json.loads()`函数用于将JSON字符串解析为Python对象,但它无法直接处理已经是Python字典对象的数据。如果您已经有一个字典对象,并且想要将它转换为JSON字符串,可以使用`json.dumps()`函数。
下面是一个示例代码,演示了如何将字典对象转换为JSON字符串:
```python
import json
# 假设这是一个字典对象
data = {
"name": "John",
"age": 30,
"city": "New York"
}
# 将字典对象转换为JSON字符串
json_str = json.dumps(data)
print(json_str)
```
在上面的代码中,我们使用`json.dumps()`函数将字典对象`data`转换为JSON字符串,并将结果打印出来。
如果您已经有一个字典对象,而不是JSON字符串,并且想要对其进行解析,您可以直接使用字典对象,而不需要使用`json.loads()`函数。例如:
```python
data = {
"name": "John",
"age": 30,
"city": "New York"
}
print(data["name"])
print(data["age"])
print(data["city"])
```
在这个示例中,我们直接使用字典对象`data`来访问特定的字段值,并打印出结果。不需要使用`json.loads()`函数来解析JSON字符串。
相关问题
TypeError: the JSON object must be str, bytes or bytearray, not TextIOWrapper
这个错误通常是因为在使用 `json.load()` 或 `json.loads()` 函数时,传入了一个 `TextIOWrapper` 类型的对象而不是一个字符串或字节流。
`json.load()` 和 `json.loads()` 函数都需要读取一个 JSON 格式的字符串或字节流来解析成 Python 对象。如果传入的是一个 `TextIOWrapper` 类型的对象,需要先调用该对象的 `read()` 方法将其转换为一个字符串或字节流,然后再传给 `json.load()` 或 `json.loads()` 函数。
示例代码:
```
import json
# 从文件中读取 JSON 格式的数据
with open('data.json', 'r') as f:
data = json.load(f)
# 从字符串中读取 JSON 格式的数据
json_str = '{"name": "Alice", "age": 20}'
data = json.loads(json_str)
```
注意:`json.load()` 函数只能从文件中读取数据,而 `json.loads()` 函数只能从字符串中读取数据。
python TypeError: the JSON object must be str, bytes or bytearray, not NoneType
This error occurs when you try to pass a `None` value to a function that expects a JSON object as a string, bytes or bytearray.
To fix this error, you need to make sure that the JSON object you are passing is not `None`. You can do this by checking the value of the object before passing it to the function.
For example, if you are using the `json.loads()` function to load a JSON object from a file, you can check if the file exists and is not empty before passing it to the function:
```
import json
with open('data.json', 'r') as f:
data = f.read()
if data:
json_data = json.loads(data)
else:
# handle empty file
```
In this example, if the `data` variable is `None`, the `json.loads()` function will raise the `TypeError` exception. However, by checking if `data` is not empty before calling the function, we can avoid this error.
相关推荐
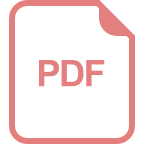
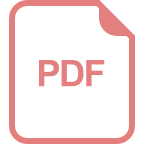
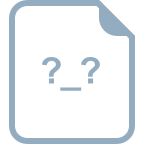









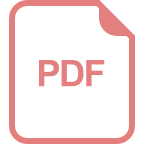
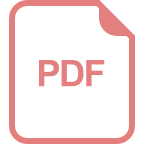
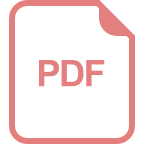
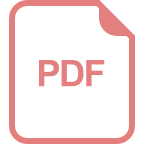