python特效代码
时间: 2024-03-08 10:44:18 浏览: 22
当提到Python的特效代码,通常指的是一些可以产生视觉或动画效果的代码。以下是一些常见的Python特效代码示例:
1. 火焰效果:
```python
import pygame
import random
pygame.init()
width, height = 800, 600
screen = pygame.display.set_mode((width, height))
clock = pygame.time.Clock()
fire_colors = [(255, 0, 0), (255, 165, 0), (255, 255, 0)]
fire_pixels = []
for _ in range(width):
fire_pixels.append([0] * height)
def update_fire():
for x in range(width):
for y in range(1, height):
decay = random.randint(0, 3)
fire_pixels[x][y - decay] = min(fire_pixels[x][y], 255)
def render_fire():
for x in range(width):
for y in range(height):
color = fire_colors[fire_pixels[x][y] // 85]
screen.set_at((x, y), color)
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
update_fire()
render_fire()
pygame.display.flip()
clock.tick(60)
pygame.quit()
```
2. 粒子效果:
```python
import pygame
import random
pygame.init()
width, height = 800, 600
screen = pygame.display.set_mode((width, height))
clock = pygame.time.Clock()
particles = []
for _ in range(100):
x = random.randint(0, width)
y = random.randint(0, height)
dx = random.uniform(-1, 1)
dy = random.uniform(-1, 1)
particles.append((x, y, dx, dy))
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((0, 0, 0))
for i in range(len(particles)):
x, y, dx, dy = particles[i]
x += dx
y += dy
particles[i] = (x, y, dx, dy)
pygame.draw.circle(screen, (255, 255, 255), (int(x), int(y)), 2)
pygame.display.flip()
clock.tick(60)
pygame.quit()
```
3. 文字动画效果:
```python
import pygame
pygame.init()
width, height = 800, 600
screen = pygame.display.set_mode((width, height))
clock = pygame.time.Clock()
font = pygame.font.Font(None, 36)
text = font.render("Hello, World!", True, (255, 255, 255))
text_rect = text.get_rect(center=(width // 2, height // 2))
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((0, 0, 0))
screen.blit(text, text_rect)
pygame.display.flip()
clock.tick(60)
pygame.quit()
```
相关推荐
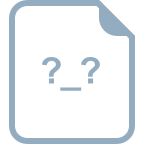














