用c语言实现cc2530芯片的无线串口通信
时间: 2023-10-23 08:11:50 浏览: 187
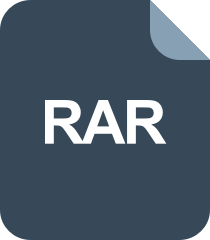
CC2530 无线串口实现

CC2530 是一种低功耗的无线 SoC 芯片,可用于 Zigbee、6LoWPAN 和 RF4CE 等无线通信应用。要在 CC2530 上实现无线串口通信,可以使用 TI 的 Z-Stack 协议栈和 CC2530 芯片的串口接口。
以下是一个基本的 C 语言代码示例,用于在 CC2530 上实现无线串口通信:
```
#include <stdio.h>
#include "hal_lcd.h"
#include "hal_uart.h"
#include "hal_board.h"
#include "OSAL.h"
#include "ZComDef.h"
#include "ZGlobals.h"
#include "AF.h"
#include "ZDApp.h"
#include "MT_SYS.h"
#include "MT_UART.h"
#include "ti_drivers_config.h"
#define RX_BUF_SIZE 128
#define TX_BUF_SIZE 128
static uint8_t rx_buf[RX_BUF_SIZE];
static uint8_t tx_buf[TX_BUF_SIZE];
static uint8_t rx_len = 0;
static uint8_t tx_len = 0;
void uart_rx_handler(uint8 port, uint8 event)
{
uint8_t c;
if (HAL_UART_RX_FULL == event)
{
HAL_UART_READ(port, &c, 1);
if (rx_len < RX_BUF_SIZE)
{
rx_buf[rx_len++] = c;
}
}
}
void uart_tx_handler(uint8 port, uint8 event)
{
if (HAL_UART_TX_EMPTY == event)
{
if (tx_len > 0)
{
HAL_UART_WRITE(port, &tx_buf[0], 1);
tx_len--;
memcpy(&tx_buf[0], &tx_buf[1], tx_len);
}
}
}
void main()
{
const uint32_t baud_rate = 9600;
// 初始化串口
halBoardInit();
halUARTCfg_t uart_cfg;
uart_cfg.configured = TRUE;
uart_cfg.baudRate = baud_rate;
uart_cfg.flowControl = HAL_UART_FLOW_OFF;
uart_cfg.dataBits = HAL_UART_LEN_8;
uart_cfg.parity = HAL_UART_PAR_NONE;
uart_cfg.stopBits = HAL_UART_STOP_BITS_1;
HalUARTInit();
// 注册串口接收和发送处理程序
MT_UartRegisterTaskID(1, 1);
MT_UartRegisterTaskID(1, 2);
HalUARTPollInit();
// 循环处理串口数据
while (1)
{
if (rx_len > 0)
{
uint8_t c = rx_buf[0];
rx_len--;
memcpy(&rx_buf[0], &rx_buf[1], rx_len);
// 处理接收到的数据
// ...
// 发送数据
if (tx_len < TX_BUF_SIZE)
{
tx_buf[tx_len++] = c;
}
}
// 检查串口发送缓冲区
HalUARTPollTxHandler(1);
}
}
```
在这个示例代码中,我们使用了 TI 的 Z-Stack 协议栈和 CC2530 芯片的 HAL 库来访问串口接口。我们定义了两个数组 rx_buf 和 tx_buf,分别用于存储接收到的数据和要发送的数据。我们还定义了两个变量 rx_len 和 tx_len,用于记录数组中有效数据的长度。
在程序的主循环中,我们使用 `HalUARTPollInit` 函数初始化串口,并使用 `MT_UartRegisterTaskID` 函数注册串口接收和发送处理程序。然后,我们不断循环检查串口接收缓冲区中是否有新数据到达,如果有,就将其存储到 rx_buf 数组中,并处理接收到的数据。如果需要发送数据,就将其存储到 tx_buf 数组中,并使用 `HalUARTPollTxHandler` 函数检查串口发送缓冲区,以确保数据尽快发送。
这个示例代码只是一个基本的框架,具体的实现细节需要根据实际情况进行调整和优化。例如,我们可以添加数据校验、错误处理等功能,以确保通信的可靠性和稳定性。
阅读全文
相关推荐
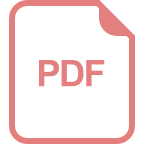

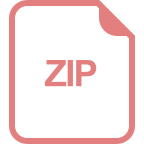
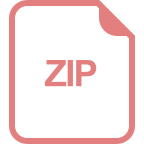
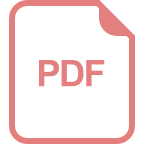
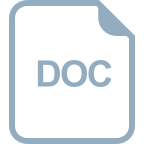
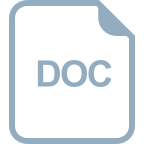
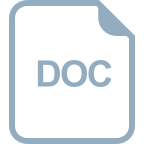
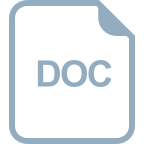
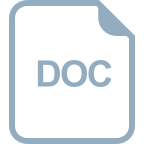
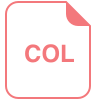


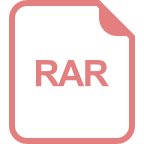
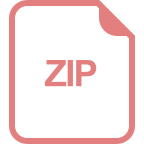
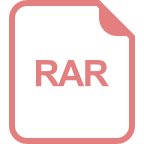
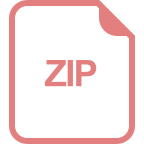