C语言中接口异步执行,不阻塞
时间: 2024-05-01 07:22:01 浏览: 111
在C语言中,要实现异步执行且不阻塞的接口,可以使用多线程或异步回调函数。
1. 使用多线程
可以使用pthread库中的pthread_create函数创建一个新线程,将需要异步执行的任务放在新线程中执行,主线程可以继续执行其他任务,不会被阻塞。
示例代码:
```c
#include <stdio.h>
#include <pthread.h>
void* async_task(void* arg) {
// 异步执行的任务
printf("Async task is running.\n");
return NULL;
}
int main() {
pthread_t thread;
int ret = pthread_create(&thread, NULL, async_task, NULL);
if (ret != 0) {
printf("Failed to create thread.\n");
return 1;
}
printf("Main thread is running.\n");
// 等待异步任务完成
pthread_join(thread, NULL);
return 0;
}
```
2. 使用异步回调函数
可以使用异步回调函数来实现异步执行,不阻塞。具体实现方式是,在调用接口时,传入一个回调函数,异步任务完成后,调用回调函数通知结果。
示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
typedef void (*async_callback)(int result);
void async_task(async_callback callback) {
// 异步执行的任务
sleep(3);
int result = rand() % 100;
callback(result);
}
void async_callback_func(int result) {
printf("Async task is finished, result = %d.\n", result);
}
int main() {
async_task(async_callback_func);
printf("Main thread is running.\n");
return 0;
}
```
在上面的代码中,async_task函数是一个异步执行的任务,它接收一个回调函数作为参数,任务完成后调用回调函数通知结果。在主函数中,调用async_task函数时传入了一个回调函数async_callback_func。这样,在异步任务完成后,会调用async_callback_func函数通知结果。在此期间,主线程可以继续执行其他任务,不会被阻塞。
阅读全文
相关推荐
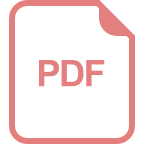
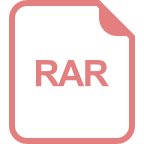
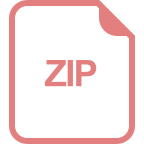
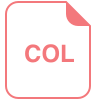
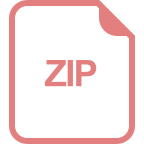
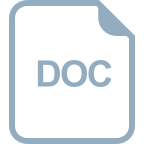
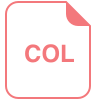

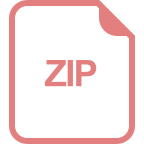
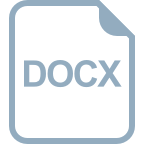
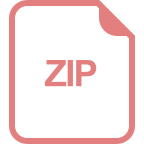
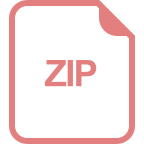
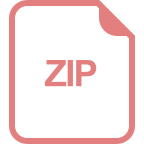
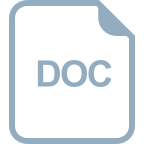
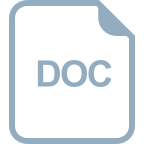
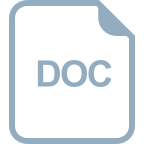
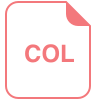
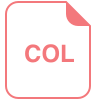