c语言中导入txt
时间: 2023-07-02 20:15:41 浏览: 788
在 C 语言中,可以使用标准库中的函数来导入 txt 文件。以下是一个简单的示例:
```c
#include <stdio.h>
int main() {
FILE *fp;
char buffer[100];
// 打开文件
fp = fopen("file.txt", "r");
// 按行读取数据
while (fgets(buffer, 100, fp) != NULL) {
printf("%s", buffer);
}
// 关闭文件
fclose(fp);
return 0;
}
```
在这个示例中,我们使用了 `fopen()` 函数来打开文件,并使用 `fgets()` 函数按行读取数据。最后,我们使用 `fclose()` 函数来关闭文件。请注意,文件名需要与当前工作目录下的文件名匹配。
相关问题
c语言中导入txt第一行为字符串,剩下的为数字怎么弄
你可以使用C标准库中的fopen和fscanf函数来读取txt文件中的内容。具体步骤如下:
1. 使用fopen函数打开txt文件,返回文件指针变量。
2. 使用fscanf函数读取第一行的字符串,可以使用"%s"格式化字符。
3. 使用fscanf函数读取剩下的数字,可以使用"%d"格式化整数。
4. 使用fclose函数关闭文件。
下面是一个示例代码:
```
#include <stdio.h>
int main() {
FILE *fp;
char str[100];
int num;
fp = fopen("data.txt", "r"); // 打开文件
if (fp == NULL) {
printf("无法打开文件\n");
return 1;
}
fscanf(fp, "%s", str); // 读取第一行字符串
printf("字符串:%s\n", str);
while (fscanf(fp, "%d", &num) != EOF) { // 逐行读取数字
printf("数字:%d\n", num);
}
fclose(fp); // 关闭文件
return 0;
}
```
假设txt文件内容如下:
```
hello world
1
2
3
```
运行上述代码,输出结果为:
```
字符串:hello
数字:1
数字:2
数字:3
```
c语言中导入txt第一行为字符串,第一列为字符串,剩下的为数字怎么弄
你可以使用C标准库中的fopen和fscanf函数来读取txt文件中的内容。具体步骤如下:
1. 使用fopen函数打开txt文件,返回文件指针变量。
2. 使用fscanf函数读取第一行的字符串,可以使用"%s"格式化字符。
3. 使用fscanf函数循环读取每一行的数据,可以使用"%s"格式化字符串和"%d"格式化整数。
4. 使用fclose函数关闭文件。
下面是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
FILE *fp;
char str[100];
char name[100];
int num1, num2, num3;
fp = fopen("data.txt", "r"); // 打开文件
if (fp == NULL) {
printf("无法打开文件\n");
return 1;
}
fscanf(fp, "%s", str); // 读取第一行字符串
printf("字符串:%s\n", str);
while (fscanf(fp, "%s %d %d %d", name, &num1, &num2, &num3) != EOF) { // 逐行读取数据
printf("姓名:%s,数值:%d, %d, %d\n", name, num1, num2, num3);
}
fclose(fp); // 关闭文件
return 0;
}
```
假设txt文件内容如下:
```
hello world
Tom 1 2 3
Jerry 4 5 6
```
运行上述代码,输出结果为:
```
字符串:hello
姓名:Tom,数值:1, 2, 3
姓名:Jerry,数值:4, 5, 6
```
阅读全文
相关推荐





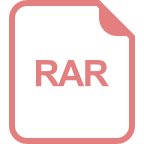










