编写程序,现有一个班4 个学生,5 门课程。键入各生各科分数,实现下列功能: ⑴求第1门课程的平均分; ⑵找出有两门以上课程不及格的学生,并输出他们的学号和全部课程成绩及平均成绩; ⑶找出平均成绩在90分以上或全部课程成绩在85分以上的学生。(文件名为sy4-2-2)用C++解决
时间: 2024-03-12 19:43:47 浏览: 57
以下是实现上述功能的 C++ 程序:
```c++
#include<iostream>
#include<fstream>
using namespace std;
const int N = 4, M = 5;
struct Student {
int scores[M];
double avg_score;
int id;
};
double calc_avg_score(Student s) {
int sum = 0;
for (int i = 0; i < M; i++) {
sum += s.scores[i];
}
s.avg_score = sum * 1.0 / M;
return s.avg_score;
}
void output(Student s) {
cout << "学号:" << s.id << " ";
cout << "成绩:";
for (int i = 0; i < M; i++) {
cout << s.scores[i] << " ";
}
cout << "平均分:" << s.avg_score << endl;
}
int main() {
Student students[N];
ifstream ifs("sy4-2-2.txt");
if (!ifs.is_open()) {
cout << "打开文件失败!" << endl;
return 0;
}
for (int i = 0; i < N; i++) {
Student s;
s.id = i + 1;
for (int j = 0; j < M; j++) {
ifs >> s.scores[j];
}
students[i] = s;
}
ifs.close();
// 求第1门课程的平均分
int sum = 0;
for (int i = 0; i < N; i++) {
sum += students[i].scores[0];
}
double avg_score_1 = sum * 1.0 / N;
cout << "第1门课程的平均分为:" << avg_score_1 << endl;
// 找出有两门以上课程不及格的学生
for (int i = 0; i < N; i++) {
int cnt = 0;
for (int j = 0; j < M; j++) {
if (students[i].scores[j] < 60) {
cnt++;
}
}
if (cnt >= 2) {
output(students[i]);
}
}
// 找出平均成绩在90分以上或全部课程成绩在85分以上的学生
for (int i = 0; i < N; i++) {
double avg_score = calc_avg_score(students[i]);
if (avg_score > 90) {
output(students[i]);
} else {
bool flag = true;
for (int j = 0; j < M; j++) {
if (students[i].scores[j] < 85) {
flag = false;
break;
}
}
if (flag) {
output(students[i]);
}
}
}
return 0;
}
```
程序首先从文件中读入学生的成绩信息,然后按照题目要求实现了三个功能:求第1门课程的平均分、找出有两门以上课程不及格的学生,并输出他们的学号和全部课程成绩及平均成绩、找出平均成绩在90分以上或全部课程成绩在85分以上的学生。程序中的 `sy4-2-2.txt` 文件中存储了学生的成绩信息,每行为一个学生的成绩,数字之间用空格分隔。
阅读全文
相关推荐
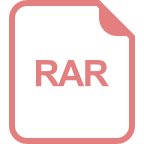
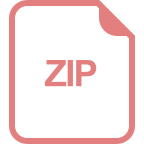
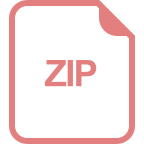
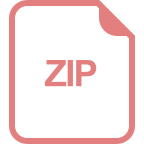
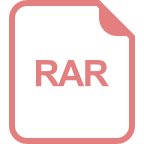
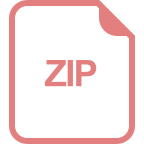
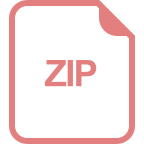
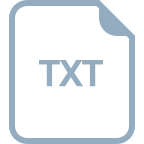
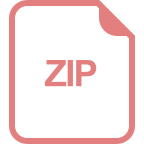
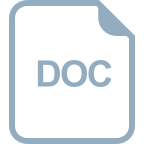
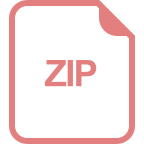
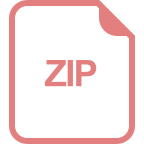
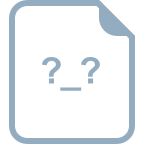
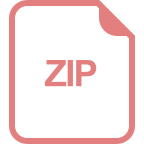
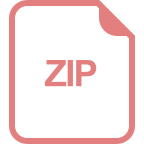
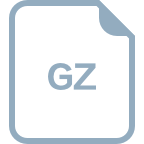
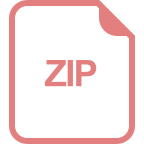
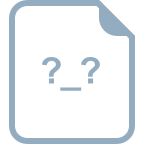