java实现rsa公钥密码
时间: 2023-10-03 08:09:05 浏览: 57
RSA 公钥密码是一种非对称加密算法,其中加密和解密使用不同的密钥。在 RSA 加密过程中,使用一个公钥对数据进行加密,但要解密该数据,则需要使用其相应的私钥。以下是 Java 实现 RSA 公钥密码的示例代码:
```java
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.PrivateKey;
import java.security.PublicKey;
import java.security.SecureRandom;
import javax.crypto.Cipher;
public class RSAEncryptionExample {
public static void main(String[] args) throws Exception {
// 生成 RSA 密钥对
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
SecureRandom secureRandom = new SecureRandom();
keyPairGenerator.initialize(2048, secureRandom);
KeyPair keyPair = keyPairGenerator.generateKeyPair();
// 获取公钥和私钥
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
String plainText = "Hello, world!";
// 使用公钥加密明文
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
byte[] encryptedMessage = cipher.doFinal(plainText.getBytes());
System.out.println("Encrypted message: " + new String(encryptedMessage));
// 使用私钥解密密文
cipher.init(Cipher.DECRYPT_MODE, privateKey);
byte[] decryptedMessage = cipher.doFinal(encryptedMessage);
System.out.println("Decrypted message: " + new String(decryptedMessage));
}
}
```
在此示例中,我们使用 `KeyPairGenerator` 生成一个 RSA 密钥对。然后我们使用公钥加密明文,并使用私钥解密密文。在加密和解密过程中,我们使用 `Cipher` 类。使用 `Cipher.getInstance("RSA")` 创建一个 RSA 加密器/解密器实例。使用 `cipher.init(Cipher.ENCRYPT_MODE, publicKey)` 初始化加密器,使用 `cipher.init(Cipher.DECRYPT_MODE, privateKey)` 初始化解密器。最后,我们将加密后的密文和解密后的明文打印到控制台上。
相关推荐
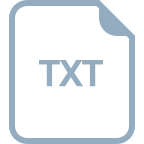
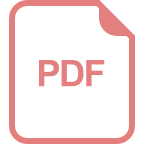
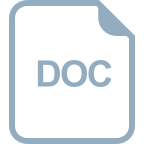














