linux下的c语言编程文件操作例题
时间: 2023-10-02 08:12:12 浏览: 118
好的,以下是一个简单的例题:
要求:编写一个程序,实现以下功能:
1. 创建一个名为test.txt的文件,并向其中写入一些内容;
2. 打开test.txt文件,读取其中的内容并输出到屏幕上;
3. 向test.txt文件中追加一些内容;
4. 再次打开test.txt文件,读取其中的内容并输出到屏幕上;
5. 关闭test.txt文件,并删除该文件。
代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
FILE *fp;
char str[100];
// 创建文件并写入内容
fp = fopen("test.txt", "w");
if (fp == NULL) {
printf("Error in creating file!\n");
exit(1);
}
fprintf(fp, "Hello World!\n");
fclose(fp);
// 读取文件内容并输出
fp = fopen("test.txt", "r");
if (fp == NULL) {
printf("Error in opening file!\n");
exit(1);
}
while (fgets(str, 100, fp) != NULL) {
printf("%s", str);
}
fclose(fp);
// 追加文件内容
fp = fopen("test.txt", "a");
if (fp == NULL) {
printf("Error in opening file!\n");
exit(1);
}
fprintf(fp, "This is a test.\n");
fclose(fp);
// 再次读取文件内容并输出
fp = fopen("test.txt", "r");
if (fp == NULL) {
printf("Error in opening file!\n");
exit(1);
}
while (fgets(str, 100, fp) != NULL) {
printf("%s", str);
}
fclose(fp);
// 删除文件
if (remove("test.txt") == 0) {
printf("File deleted successfully!\n");
} else {
printf("Error in deleting file!\n");
}
return 0;
}
```
注意:在Linux系统下,文件路径使用斜杠(/)作为分隔符,而不是反斜杠(\)。此外,需要注意文件读写权限问题。
阅读全文
相关推荐
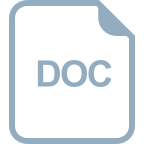
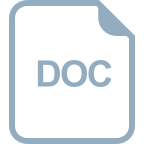
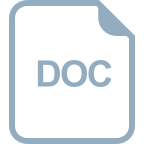
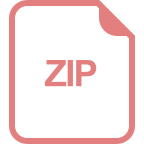
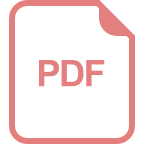
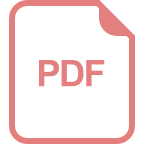
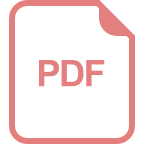
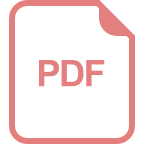
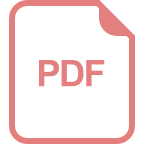
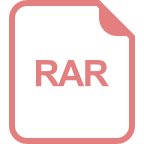
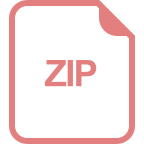
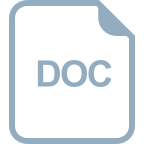
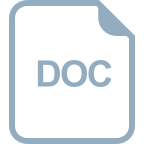
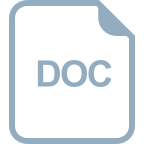
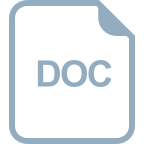
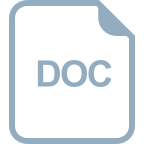


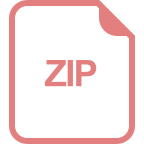