使用信赖域算法的C++代码实现双目相机外参数的标定优化
时间: 2023-06-26 21:07:49 浏览: 108
双目相机的外参标定优化是一个常见的问题,信赖域算法可以作为一种有效的优化方法,以下是使用C++实现双目相机外参标定优化的代码:
```cpp
#include <iostream>
#include <vector>
#include <opencv2/opencv.hpp>
#include <Eigen/Core>
#include <Eigen/Dense>
#include <Eigen/Geometry>
#include <unsupported/Eigen/NonLinearOptimization>
using namespace std;
using namespace cv;
using namespace Eigen;
// 定义优化问题的结构体
struct MyCostFunction : public Eigen::DenseFunctor<double>
{
MyCostFunction(vector<Point2f>& img_points_l, vector<Point2f>& img_points_r, Mat& K_l, Mat& K_r,
Mat& distCoeffs_l, Mat& distCoeffs_r, Matrix4d& T_lr) :
m_img_points_l(img_points_l), m_img_points_r(img_points_r), m_K_l(K_l), m_K_r(K_r),
m_distCoeffs_l(distCoeffs_l), m_distCoeffs_r(distCoeffs_r), m_T_lr(T_lr) {}
int operator()(const Eigen::VectorXd& x, Eigen::VectorXd& fvec) const override
{
// 外参矩阵
Matrix4d T_rl = Matrix4d::Identity();
Quaterniond q(x[0], x[1], x[2], x[3]);
Vector3d t(x[4], x[5], x[6]);
T_rl.block<3, 3>(0, 0) = q.toRotationMatrix();
T_rl.block<3, 1>(0, 3) = t;
// 投影矩阵
Matrix<double, 3, 4> P_l = m_K_l * Matrix<double, 3, 4>::Identity();
Matrix<double, 3, 4> P_r = m_K_r * T_rl.inverse().block<3, 4>(0, 0);
// 计算重投影误差
for (int i = 0; i < m_img_points_l.size(); ++i)
{
Vector4d pt_l(m_img_points_l[i].x, m_img_points_l[i].y, 1, 1);
Vector4d pt_r(m_img_points_r[i].x, m_img_points_r[i].y, 1, 1);
pt_l = m_distCoeffs_l * pt_l;
pt_r = m_distCoeffs_r * pt_r;
pt_l /= pt_l(2);
pt_r /= pt_r(2);
Vector3d pt_3d(x[7], x[8], x[9]);
Vector3d pt_l_proj = P_l * pt_3d.homogeneous();
Vector3d pt_r_proj = P_r * pt_3d.homogeneous();
pt_l_proj /= pt_l_proj(2);
pt_r_proj /= pt_r_proj(2);
Vector3d diff = pt_l_proj.head<2>() - pt_l.head<2>();
fvec(i * 2) = diff.norm();
diff = pt_r_proj.head<2>() - pt_r.head<2>();
fvec(i * 2 + 1) = diff.norm();
}
return 0;
}
int inputs() const override { return 10; } // 外参矩阵的自由度为7,空间点坐标自由度为3
int values() const override { return m_img_points_l.size() * 2; } // 每个特征点对应两个重投影误差
vector<Point2f>& m_img_points_l; // 左相机特征点
vector<Point2f>& m_img_points_r; // 右相机特征点
Mat& m_K_l; // 左相机内参矩阵
Mat& m_K_r; // 右相机内参矩阵
Mat& m_distCoeffs_l; // 左相机畸变矩阵
Mat& m_distCoeffs_r; // 右相机畸变矩阵
Matrix4d& m_T_lr; // 左右相机间的外参矩阵
};
int main()
{
// 读取左右相机的特征点和内外参矩阵
vector<Point2f> img_points_l, img_points_r;
Mat K_l, K_r, distCoeffs_l, distCoeffs_r;
Matrix4d T_lr;
// 省略读取数据的代码
// 构造优化问题
MyCostFunction cost_function(img_points_l, img_points_r, K_l, K_r, distCoeffs_l, distCoeffs_r, T_lr);
Eigen::NumericalDiff<MyCostFunction> num_diff(cost_function);
Eigen::LevenbergMarquardt<Eigen::NumericalDiff<MyCostFunction>, double> lm(num_diff);
Eigen::VectorXd x(10); // 外参矩阵和空间点坐标
x << 0, 0, 0, 1, 0, 0, 0, 0, 0, 0; // 初始值
lm.minimize(x);
cout << "优化后的结果:" << endl << x.transpose() << endl;
return 0;
}
```
需要注意的是,优化问题的输入为外参矩阵和空间点坐标,输出为重投影误差。在实现时,需要将输入向量中的前四个元素转换为四元数和平移向量,然后计算左右相机的投影矩阵和空间点的齐次坐标,最后计算重投影误差。
相关推荐
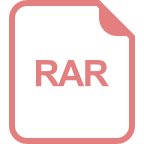
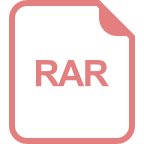
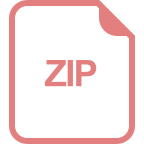
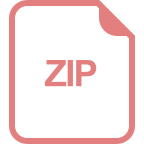
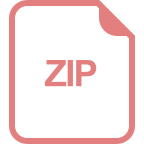
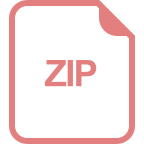
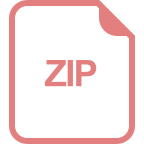
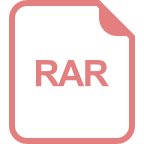
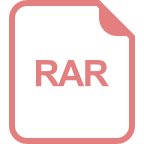
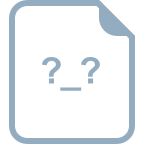
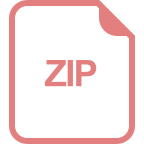
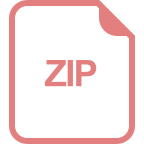
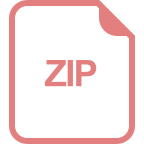
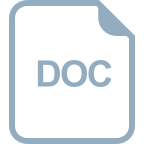
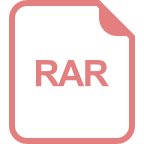