如何用python ,利用中文分词jieba库,统计一篇txt中文文章中出现频率最高的10个中文词。
时间: 2024-02-28 16:53:49 浏览: 100
可以按照以下步骤使用Python和jieba库来实现:
1. 安装jieba库,可以使用以下命令:
```
pip install jieba
```
2. 读取要分析的中文文本文件,可以使用Python内置的open()函数来打开文件。
```
with open('your_file.txt', 'r', encoding='utf-8') as f:
text = f.read()
```
3. 使用jieba库进行中文分词,可以使用jieba.cut()方法。
```
import jieba
words = jieba.cut(text)
```
4. 统计分词后的词语出现次数,可以使用Python内置的collections.Counter()方法。
```
from collections import Counter
word_counts = Counter(words)
```
5. 找出出现频率最高的10个中文词,并输出结果。
```
top_words = word_counts.most_common(10)
for word, count in top_words:
print(word, count)
```
完整代码如下:
```
import jieba
from collections import Counter
with open('your_file.txt', 'r', encoding='utf-8') as f:
text = f.read()
words = jieba.cut(text)
word_counts = Counter(words)
top_words = word_counts.most_common(10)
for word, count in top_words:
print(word, count)
```
阅读全文
相关推荐
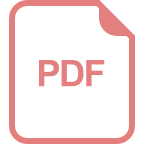
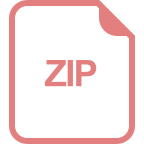
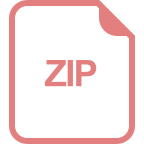














