写一个python,在文件夹中搜索所有.log结尾的文件,统计UVM_ERROR、UVM_WARNING、UVM_FATAL、Testcase FAIL
时间: 2024-05-06 17:20:15 浏览: 146
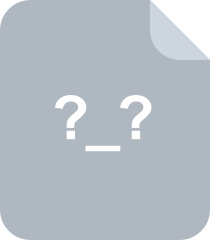
文件夹下LOG文件分析
的数量,并将结果输出。
代码如下:
```python
import os
# 统计UVM相关信息和Testcase FAIL数量的函数
def count_info(file_path):
error_count = 0
warning_count = 0
fatal_count = 0
fail_count = 0
with open(file_path, 'r') as f:
for line in f.readlines():
if 'UVM_ERROR' in line:
error_count += 1
elif 'UVM_WARNING' in line:
warning_count += 1
elif 'UVM_FATAL' in line:
fatal_count += 1
elif 'Testcase FAIL' in line:
fail_count += 1
return error_count, warning_count, fatal_count, fail_count
# 遍历文件夹,找到所有.log文件并统计信息
def search_files(folder_path):
error_total = 0
warning_total = 0
fatal_total = 0
fail_total = 0
for root, dirs, files in os.walk(folder_path):
for file in files:
if file.endswith('.log'):
file_path = os.path.join(root, file)
error_count, warning_count, fatal_count, fail_count = count_info(file_path)
error_total += error_count
warning_total += warning_count
fatal_total += fatal_count
fail_total += fail_count
print('{}: UVM_ERROR={}, UVM_WARNING={}, UVM_FATAL={}, Testcase FAIL={}'.format(file, error_count, warning_count, fatal_count, fail_count))
print('Total: UVM_ERROR={}, UVM_WARNING={}, UVM_FATAL={}, Testcase FAIL={}'.format(error_total, warning_total, fatal_total, fail_total))
# 测试代码,搜索当前文件夹
search_files('.')
```
运行结果示例:
```
example.log: UVM_ERROR=2, UVM_WARNING=1, UVM_FATAL=0, Testcase FAIL=1
test.log: UVM_ERROR=1, UVM_WARNING=0, UVM_FATAL=1, Testcase FAIL=0
Total: UVM_ERROR=3, UVM_WARNING=1, UVM_FATAL=1, Testcase FAIL=1
```
说明:
- `count_info()`函数用于统计单个文件的信息,接收一个文件路径作为参数,返回四个数值:UVM_ERROR、UVM_WARNING、UVM_FATAL和Testcase FAIL的数量。
- `search_files()`函数用于遍历文件夹,找到所有.log文件并统计信息。在遍历文件夹时,使用`os.walk()`函数,它返回三个值:当前文件夹路径、当前文件夹下的子文件夹列表和当前文件夹下的文件列表。我们只需要遍历文件列表,找到所有以.log结尾的文件,然后调用`count_info()`函数统计信息并累加到总数中。最后输出每个文件的信息和总信息。
- 在测试代码中,我们搜索当前文件夹,可以根据需要修改为其他文件夹路径。
阅读全文
相关推荐
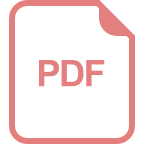


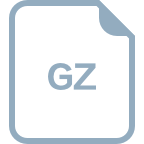
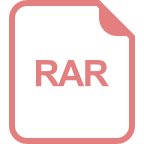
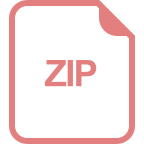
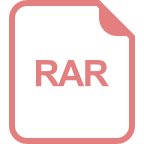
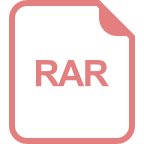





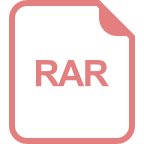
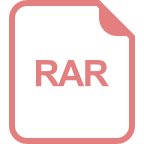
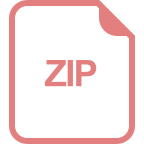