用python写一个可以在网页运行的小游戏
时间: 2023-06-14 17:04:55 浏览: 58
好的,我可以给你一个简单的例子,用Python Flask框架写一个猜数字的小游戏。
首先,你需要安装 Flask。可以在命令行中输入以下命令安装:
```python
pip install Flask
```
然后,可以在项目文件夹中创建一个名为 `app.py` 的文件,并将以下代码复制到文件中:
```python
from flask import Flask, render_template, request, session
import random
app = Flask(__name__)
app.secret_key = 'your_secret_key'
@app.route('/', methods=['GET', 'POST'])
def index():
if request.method == 'POST':
session['guess'] = int(request.form['guess'])
return render_template('result.html')
session['number'] = random.randint(1, 100)
return render_template('index.html')
@app.route('/result')
def result():
guess = session['guess']
number = session['number']
if guess == number:
result = 'Congratulations! You guessed the number.'
elif guess > number:
result = 'Too high! Try again.'
else:
result = 'Too low! Try again.'
return render_template('result.html', result=result)
if __name__ == '__main__':
app.run(debug=True)
```
这个应用程序有三个路由:
- `/`:显示主页,让用户猜一个 1 到 100 之间的数字。
- `/result`:处理用户提交的猜测,并显示结果页面。
- `/static`:提供静态文件(CSS,JavaScript等)。
在项目文件夹中创建一个名为 `templates` 的文件夹,并将以下代码复制到 `index.html` 文件中:
```html
<!DOCTYPE html>
<html>
<head>
<title>Guess the number</title>
<link href="{{ url_for('static', filename='style.css') }}" rel="stylesheet">
</head>
<body>
<h1>Guess the number</h1>
<form method="POST" action="/">
<p>Guess the number between 1 and 100:</p>
<input type="number" name="guess" required>
<button type="submit">Submit</button>
</form>
</body>
</html>
```
然后,在 `templates` 文件夹中创建一个名为 `result.html` 的文件,并将以下代码复制到文件中:
```html
<!DOCTYPE html>
<html>
<head>
<title>Guess the number</title>
<link href="{{ url_for('static', filename='style.css') }}" rel="stylesheet">
</head>
<body>
<h1>Guess the number</h1>
<p>{{ result }}</p>
<form method="POST" action="/">
<button type="submit">Play again</button>
</form>
</body>
</html>
```
最后,在项目文件夹中创建一个名为 `style.css` 的文件,并将以下代码复制到文件中:
```css
body {
font-family: Arial, sans-serif;
text-align: center;
}
h1 {
color: #333;
}
button {
background-color: #4CAF50;
border: none;
color: white;
padding: 10px 20px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 4px 2px;
cursor: pointer;
}
input[type=number] {
width: 40px;
}
```
现在,你可以在命令行中运行 `app.py` 文件,然后在浏览器中访问 `http://127.0.0.1:5000/` 来玩这个小游戏了。
希望这个例子能帮到你,让你了解如何用 Python Flask 框架写一个简单的网页游戏。
相关推荐
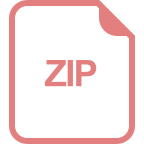
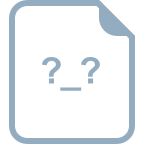
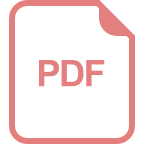









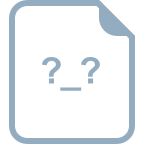
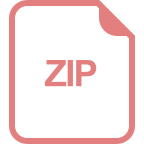
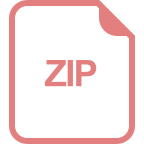
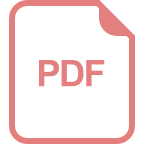
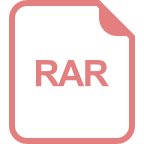
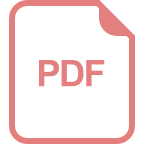
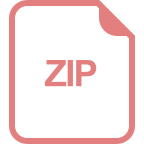