SpringBoot+Thymeleaf中controller修改方法怎么写
时间: 2024-01-21 21:17:09 浏览: 29
在SpringBoot+Thymeleaf中,Controller中的修改方法通常需要以下步骤:
1. 在Controller中定义一个处理修改请求的方法,例如:
```java
@RequestMapping(value = "/edit/{id}", method = RequestMethod.POST)
public String edit(@PathVariable("id") Long id, Model model, @ModelAttribute("form") MyForm form) {
// 根据id查询要修改的数据
MyData myData = myDataService.findById(id);
if (myData == null) {
throw new NotFoundException();
}
// 将form中的数据赋值给myData
myData.setName(form.getName());
myData.setValue(form.getValue());
// 更新数据
myDataService.save(myData);
// 重定向到列表页面
return "redirect:/list";
}
```
2. 在Thymeleaf模板中,使用form表单提交修改请求,例如:
```html
<form th:action="@{/edit/{id}(id=${myData.id})}" method="post" th:object="${form}">
<div class="form-group">
<label for="name">Name</label>
<<input type="text" class="form-control" id="name" name="name" th:value="*{name}" />
</div>
<div class="form-group">
<label for="value">Value</label>
<input type="text" class="form-control" id="value" name="value" th:value="*{value}" />
</div>
<button type="submit" class="btn btn-primary">Save</button>
</form>
```
3. 在Controller中,需要将修改前的数据显示在修改页面上,例如:
```java
@RequestMapping(value = "/edit/{id}", method = RequestMethod.GET)
public String edit(@PathVariable("id") Long id, Model model) {
// 根据id查询要修改的数据
MyData myData = myDataService.findById(id);
if (myData == null) {
throw new NotFoundException();
}
// 将数据传递给form对象
MyForm form = new MyForm();
form.setName(myData.getName());
form.setValue(myData.getValue());
// 将form对象传递给页面
model.addAttribute("form", form);
return "edit";
}
```
这样,在访问修改页面时,会将修改前的数据显示在表单中,用户可以直接修改并提交。
相关推荐
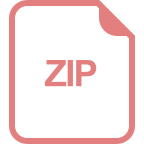
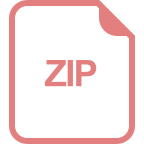
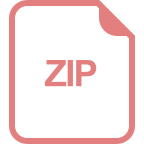














