all_theta.extend(theta)
时间: 2023-06-19 13:06:57 浏览: 148
这行代码的作用是将一个列表 theta 中的元素添加到另一个列表 all_theta 的末尾。具体来说,它会将 theta 中的每个元素添加到 all_theta 中,扩展 all_theta 的长度。注意,extend() 方法不会创建新的列表,它只是修改现有的列表。因此,如果你希望保留原始列表,可以在调用 extend() 方法之前先创建 all_theta 的副本。
相关问题
theta = atan2(sample(1)-closestNode(1),sample(2)-closestNode(2)); % 方向扩展样品以产生新的节点 direction to extend sample to produce new node newPoint = double(int32(closestNode(1:2) + stepsize * [sin(theta) cos(theta)])); if ~checkPath(closestNode(1:2), newPoint, map) % 树中最近节点向新点的扩展是否可行 if extension of closest node in tree to the new point is feasible failedAttempts = failedAttempts + 1; continue; end
这段代码是RRT(Rapidly-exploring Random Tree)算法中的一部分,用于扩展树结构,生成新的树节点。其中,sample是随机采样的点,closestNode是树中距离该点最近的节点,stepsize是步长,map是地图信息。
首先通过计算两点之间的方向角theta,确定从closestNode到sample的方向,然后将stepsize沿该方向进行扩展,得到新的节点newPoint。接着调用checkPath函数,判断从closestNode到newPoint的路径是否可行,如果可行,则将newPoint作为树中closestNode的子节点,否则继续采样新的点,直到找到可行的路径或者达到最大尝试次数。
RRT算法是一种用于路径规划的随机采样算法,其基本思想是通过不断扩展树结构,生成一棵包含起点和终点的树,最终从树中找到一条可行的路径。RRT算法具有简单、高效、可扩展性强等优点,在机器人路径规划、游戏AI等领域得到广泛应用。
阅读全文
相关推荐
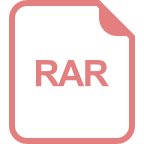
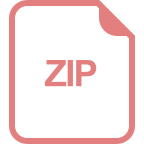
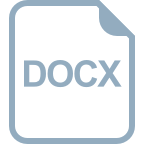
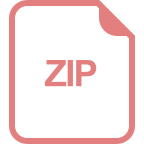
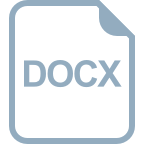
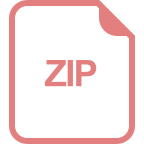
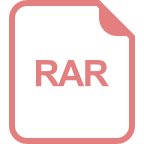
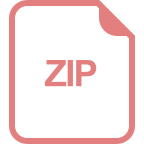
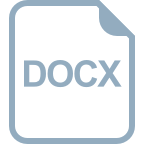
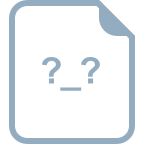
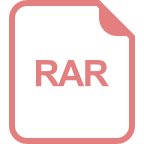
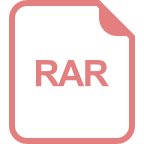
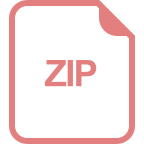