请帮我写一个python代码实现BP神经网络多维时间序列数据输入预测多维数据输出
时间: 2023-12-28 15:05:34 浏览: 46
好的,下面是一个简单的BP神经网络多维时间序列数据输入预测多维数据输出的Python代码示例:
```python
import numpy as np
class BPNeuralNetwork:
def __init__(self, input_dim, hidden_dim, output_dim):
self.input_dim = input_dim
self.hidden_dim = hidden_dim
self.output_dim = output_dim
self.weights1 = np.random.randn(hidden_dim, input_dim)
self.bias1 = np.random.randn(hidden_dim, 1)
self.weights2 = np.random.randn(output_dim, hidden_dim)
self.bias2 = np.random.randn(output_dim, 1)
def sigmoid(self, x):
return 1 / (1 + np.exp(-x))
def sigmoid_derivative(self, x):
return x * (1 - x)
def forward(self, x):
self.z1 = np.dot(self.weights1, x) + self.bias1
self.a1 = self.sigmoid(self.z1)
self.z2 = np.dot(self.weights2, self.a1) + self.bias2
self.a2 = self.sigmoid(self.z2)
return self.a2
def backward(self, x, y, output):
error = output - y
delta2 = error * self.sigmoid_derivative(output)
d_weights2 = np.dot(delta2, self.a1.T)
d_bias2 = delta2
delta1 = np.dot(self.weights2.T, delta2) * self.sigmoid_derivative(self.a1)
d_weights1 = np.dot(delta1, x.T)
d_bias1 = delta1
return d_weights1, d_bias1, d_weights2, d_bias2
def train(self, x_train, y_train, epochs=1000, learning_rate=0.1):
for epoch in range(epochs):
for i in range(len(x_train)):
x = x_train[i].reshape(-1, 1)
y = y_train[i].reshape(-1, 1)
output = self.forward(x)
d_weights1, d_bias1, d_weights2, d_bias2 = self.backward(x, y, output)
self.weights1 -= learning_rate * d_weights1
self.bias1 -= learning_rate * d_bias1
self.weights2 -= learning_rate * d_weights2
self.bias2 -= learning_rate * d_bias2
if epoch % 100 == 0:
loss = np.mean(np.square(y_train - self.forward(x_train.T)))
print(f"Epoch {epoch}. Loss: {loss}")
def predict(self, x_test):
return self.forward(x_test.T)
```
使用方法:
```python
# 生成训练数据
x_train = np.array([[0.1, 0.2, 0.3],
[0.2, 0.3, 0.4],
[0.3, 0.4, 0.5],
[0.4, 0.5, 0.6],
[0.5, 0.6, 0.7]])
y_train = np.array([[0.4, 0.5],
[0.5, 0.6],
[0.6, 0.7],
[0.7, 0.8],
[0.8, 0.9]])
# 创建神经网络
input_dim = 3
hidden_dim = 4
output_dim = 2
bpnn = BPNeuralNetwork(input_dim, hidden_dim, output_dim)
# 训练神经网络
bpnn.train(x_train, y_train, epochs=1000, learning_rate=0.1)
# 使用神经网络进行预测
x_test = np.array([[0.6, 0.7, 0.8]])
y_pred = bpnn.predict(x_test)
print(y_pred)
```
阅读全文
相关推荐
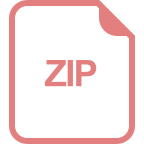
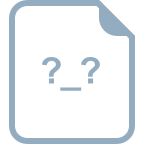
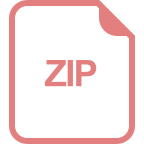

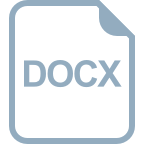
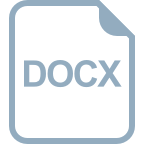
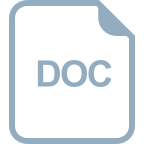

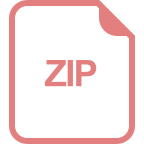
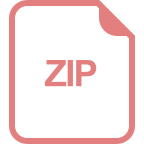
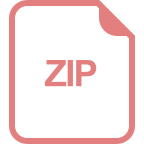
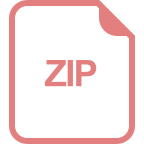
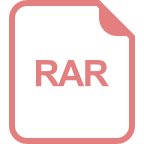
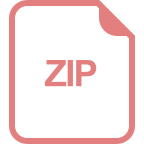