@SuppressLint("Range") public JSONArray selectApms(Long sceneStartTime) { JSONArray apmJsonArray = new JSONArray(); Cursor cursor = mRDB.query(TABLE_PERFORMANCE, null, "scene_start_time=?", new String[]{String.valueOf(sceneStartTime)}, null, null, "_id", null); if (cursor.getCount() > 0) { while (cursor.moveToNext()) { ApmEntity apmEntity = new ApmEntity(); cursor.getLong(cursor.getColumnIndex("_id")); apmEntity.setSceneStartTime(cursor.getLong(cursor.getColumnIndex( "scene_start_time"))); apmEntity.setTs(cursor.getLong(cursor.getColumnIndex("ts"))); apmEntity.setPss(cursor.getString(cursor.getColumnIndex("pss"))); apmEntity.setCpu(cursor.getString(cursor.getColumnIndex("cpu"))); apmEntity.setCurrent(cursor.getString(cursor.getColumnIndex("current"))); apmEntity.setVoltage(cursor.getString(cursor.getColumnIndex("voltage"))); apmEntity.setBatteryPercent(cursor.getString(cursor.getColumnIndex( "battery_percent"))); apmJsonArray.put(apmEntity); } return apmJsonArray; } return apmJsonArray; }
时间: 2024-02-14 10:07:45 浏览: 245
以下是一些可能的优化建议:
1. 对于查询语句,应该尽量避免使用 SELECT *,而是仅选择所需的列。这可以提高查询性能并减少内存使用量。
2. 可以使用 try-with-resources 语句来确保在完成后关闭 Cursor 对象。这可以避免内存泄漏和其他问题。
3. 在使用 Cursor 时,建议使用 getColumnIndex() 方法来获取列的索引,而不是硬编码列名。这可以提高代码的可读性和可维护性。
4. 由于 JSONArray 和 ApmEntity 之间的转换可能会导致性能问题,可以考虑直接使用 Cursor 对象来处理数据。这可以减少内存使用量并提高代码执行速度。
5. 如果查询结果集较大,可以考虑使用分页查询来减少内存使用量。可以使用 LIMIT 和 OFFSET 子句来实现分页查询。
6. 可以考虑添加索引来加速查询,例如在 scene_start_time 列上添加一个索引。
综上所述,以下是一个可能的优化版本的代码:
```
@SuppressLint("Range")
public Cursor selectApms(Long sceneStartTime) {
String[] columns = {"_id", "scene_start_time", "ts", "pss", "cpu", "current", "voltage", "battery_percent"};
String selection = "scene_start_time=?";
String[] selectionArgs = {String.valueOf(sceneStartTime)};
String orderBy = "_id";
return mRDB.query(TABLE_PERFORMANCE, columns, selection, selectionArgs, null, null, orderBy, null);
}
```
阅读全文
相关推荐
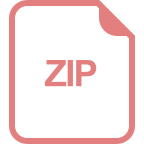
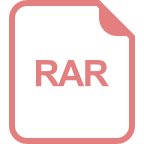
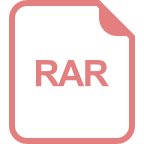















