用c++写一个单例模式
时间: 2024-02-18 17:54:42 浏览: 18
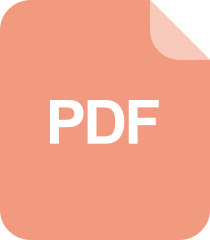
C++中的单例模式介绍
单例模式是指在整个程序中,某个类只有一个实例,且该实例易于外界访问,从而方便对整个系统的数据共享。
下面是一个 C 语言实现单例模式的代码示例:
```
#include <stdio.h>
#include <pthread.h>
pthread_mutex_t lock;
typedef struct {
int id;
} Singleton;
Singleton* singleton = NULL;
Singleton* getInstance() {
if (singleton == NULL) {
pthread_mutex_lock(&lock);
if (singleton == NULL) {
singleton = (Singleton*) malloc(sizeof(Singleton));
singleton->id = 0;
}
pthread_mutex_unlock(&lock);
}
return singleton;
}
int main() {
pthread_mutex_init(&lock, NULL);
Singleton* s1 = getInstance();
Singleton* s2 = getInstance();
printf("s1->id = %d\n", s1->id);
printf("s2->id = %d\n", s2->id);
s1->id = 100;
printf("s1->id = %d\n", s1->id);
printf("s2->id = %d\n", s2->id);
return 0;
}
```
在这个代码中,通过使用 `pthread_mutex_lock` 和 `pthread_mutex_unlock` 来实现线程同步,保证单例模式的唯一性。
阅读全文
相关推荐
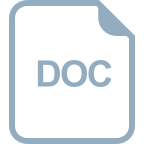
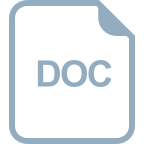



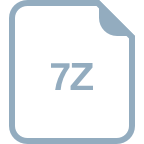

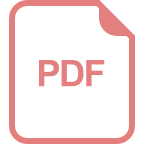





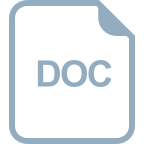
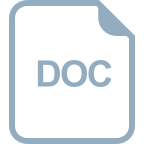