C++ shared_ptr 单例模式
时间: 2023-12-29 08:04:19 浏览: 200
C++中使用`shared_ptr`实现单例模式的方法如下所示:
```cpp
class Singleton {
private:
Singleton() {} // 私有的构造函数
public:
static std::shared_ptr<Singleton> GetInstance() {
static std::shared_ptr<Singleton> instance(new Singleton());
return instance;
}
};
```
在这个例子中,`GetInstance()`函数返回一个指向`Singleton`类对象的`shared_ptr`。通过将构造函数设为私有,我们确保只能通过`GetInstance()`函数来获取`Singleton`类的实例。同时,由于`shared_ptr`使用引用计数来管理内存,当没有其他指针指向`Singleton`实例时,内存会自动释放。
使用`shared_ptr`实现单例模式的好处是,它能够自动管理内存,避免内存泄漏的问题。此外,`shared_ptr`还提供了线程安全的引用计数,可以在多线程环境下使用。
相关问题
给出一个c++的,线程安全的,用shared_ptr封装的单例类的代码示例
当然可以,以下是一个线程安全的、使用 shared_ptr 封装的单例类的示例代码:
```c++
#include <memory>
#include <mutex>
class Singleton {
public:
static Singleton& getInstance() {
static std::shared_ptr<Singleton> instance;
static std::mutex mutex;
std::lock_guard<std::mutex> lock(mutex);
if (!instance) {
instance.reset(new Singleton);
}
return *instance;
}
void doSomething() {
// do something
}
private:
Singleton() = default;
~Singleton() = default;
Singleton(const Singleton&) = delete;
Singleton& operator=(const Singleton&) = delete;
};
```
这个示例中,我们使用了一个静态的 shared_ptr 对象来保存单例实例。在 getInstance() 方法中,我们使用了一个互斥锁来保证线程安全,并在第一次调用时创建单例实例。最后,我们返回 shared_ptr 对象的引用,以确保单例实例不会被销毁。
std::shared_ptr<AudioDevice> AudioDevice::GetInstance()
`std::shared_ptr<AudioDevice> AudioDevice::GetInstance()` 看起来像是C++中实现单例模式的一种方法,它使用了智能指针`std::shared_ptr`来管理`AudioDevice`对象的生命周期。这种实现方式确保了`AudioDevice`类只有一个实例,并且当没有任何对象需要使用这个实例时,它会被自动释放。
以下是一个简单的例子,展示了如何使用`std::shared_ptr`来实现单例模式:
```cpp
class AudioDevice {
public:
static std::shared_ptr<AudioDevice> GetInstance() {
static std::shared_ptr<AudioDevice> instance(new AudioDevice());
return instance;
}
// 由于有全局访问点,所以构造函数应为私有
private:
AudioDevice() {} // 私有构造函数防止通过其他方式创建实例
// 禁用拷贝构造函数和赋值操作符,以防止通过std::shared_ptr意外复制
AudioDevice(const AudioDevice&);
AudioDevice& operator=(const AudioDevice&);
};
// 使用
auto audioDevice = AudioDevice::GetInstance();
```
在这个例子中,`GetInstance`方法返回了一个`std::shared_ptr`,它负责管理`AudioDevice`的生命周期。使用了局部静态变量(`static std::shared_ptr<AudioDevice> instance(new AudioDevice());`)来确保无论调用`GetInstance`多少次,都只会创建一个`AudioDevice`实例。由于`std::shared_ptr`会自动处理引用计数,因此当最后一个`std::shared_ptr`被销毁时,`AudioDevice`实例也会随之被销毁。
阅读全文
相关推荐
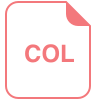
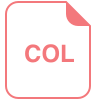
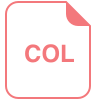
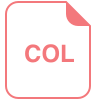
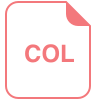
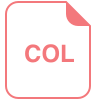
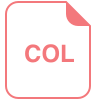

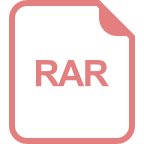
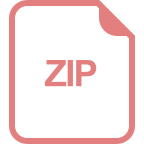
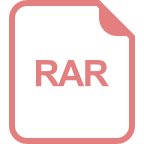
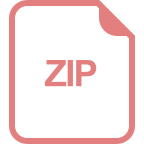
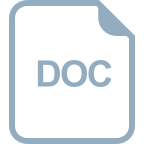
