snake->x = (snake->next->x + x) % MAP_WIDTH; snake->y = (snake->next->y + y)% MAP_HEIGHT; for (node = snake->next; node != snake; node=node->next) { node->y = node->prev->y; node->x = node->prev->x;
时间: 2024-04-26 12:24:02 浏览: 57
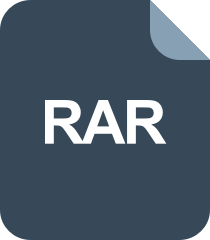
snake算法

这段代码实现了蛇的移动功能,snake表示链表的头结点,x和y分别表示横向和纵向的移动距离。具体实现方法是:
1. 先更新头结点的位置,将头结点的位置设置为原来的下一个节点的位置加上移动距离,同时对地图宽度和高度取模,以防止蛇越过地图边界。
2. 遍历链表,从蛇的第二个节点开始,将每个节点的位置设置为它前一个节点的位置,这样就实现了蛇身体的移动效果。
需要注意的是,这段代码可能存在的问题是:
1. 没有对链表是否为空进行判断,如果链表为空则会导致程序崩溃。
2. 没有对链表中只有一个节点的情况进行特殊处理,因为只有一个节点的时候,该节点的prev指针为空,可能会导致程序崩溃。
3. 没有对节点位置的边界进行判断,如果节点位置超出了屏幕边界,可能会导致蛇跑出屏幕。
4. 没有对节点的数据结构进行说明,可能会导致其他人在阅读代码时理解困难。
因此,在实际应用中,需要根据具体情况进行细节的处理和完善。
阅读全文
相关推荐
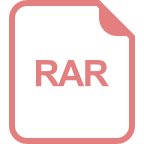
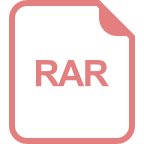
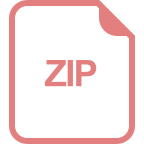
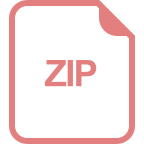
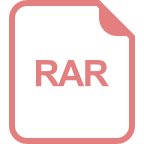
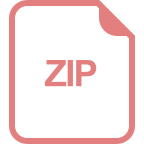
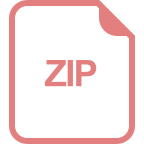
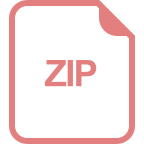
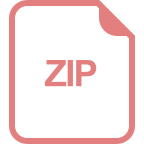
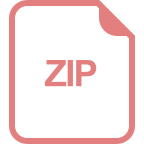
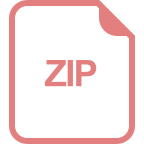
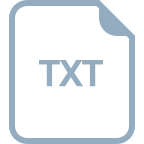