运用vue过渡和动画的知识,使用animte.css实现动画,要求实现一张图片的动态显示和隐藏,要求: 1.动画时长2s; 2.动画播放次数两次: 3.在页面上显示出自己的班级学号姓名。
时间: 2023-11-22 21:55:42 浏览: 105
以下是使用Vue过渡和动画以及Animate.css实现图片动态显示和隐藏的代码:
```html
<template>
<div>
<h1>{{name}} {{classNum}}</h1>
<button @click="showImage = !showImage">Toggle Image</button>
<transition name="fade" :duration="{ enter: 2000, leave: 2000 }" mode="out-in" :appear="true">
<img v-if="showImage" src="./path/to/image.png" alt="An image" class="animated" :class="{'bounceIn': showImage, 'bounceOut': !showImage}" @animationend="animationEnded">
</transition>
</div>
</template>
<script>
import 'animate.css';
export default {
name: 'ImageAnimation',
data() {
return {
showImage: false,
name: 'Your Name',
classNum: 'Your Class Number'
};
},
methods: {
animationEnded() {
if (!this.showImage) {
this.$nextTick(() => {
this.showImage = true;
});
}
}
}
};
</script>
<style scoped>
.fade-enter-active,
.fade-leave-active {
transition: opacity 2s;
}
.fade-enter,
.fade-leave-to {
opacity: 0;
}
.bounceIn,
.bounceOut {
animation-duration: 2s;
animation-iteration-count: 2;
}
.bounceOut {
animation-direction: reverse;
}
</style>
```
解释一下代码:
1. 在`template`中,首先展示了姓名和班级号,然后有一个按钮用于切换图片的显示状态。
2. 使用Vue的`<transition>`组件来包装图片元素,并使用`name`属性为其命名。
3. `duration`属性用于设置动画时长,`mode`属性设置为`out-in`,表示旧元素先进行离开动画,然后新元素进行进入动画,`appear`属性设置为`true`表示组件第一次渲染时也会进行过渡动画。
4. 图片元素使用`v-if`指令来控制其显示或隐藏,`class`属性绑定了一个对象,根据`showImage`的值来决定是添加`bounceIn`还是`bounceOut`类,这两个类分别对应显示和隐藏动画。
5. `@animationend`事件用于监听动画结束事件,当动画结束时,如果`showImage`为`false`,则将其设置为`true`,这样在下一次切换时,可以再次播放动画。
6. 在`<style>`标签中,使用了`scoped`属性来限制样式只在当前组件中生效。`.fade-enter-active`和`.fade-leave-active`用于设置过渡动画的样式,`.fade-enter`和`.fade-leave-to`分别对应进入和离开动画的状态。`.bounceIn`和`.bounceOut`是Animate.css提供的类,用于实现动画效果。
希望这段代码能够帮助你实现想要的动画效果。
阅读全文
相关推荐
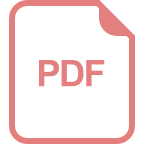

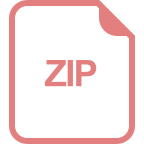
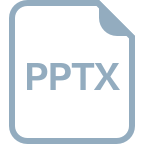
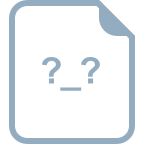
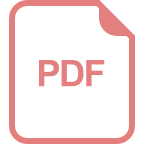
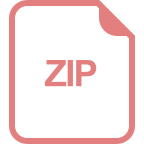
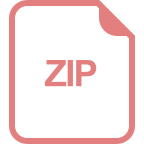
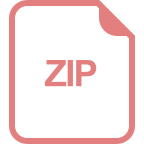
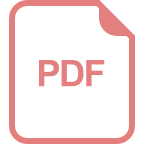
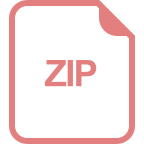
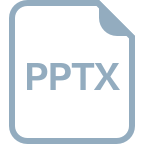
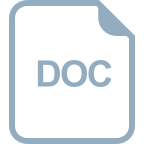
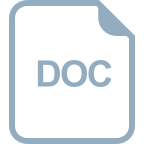