用函数实现选择或冒泡排序:定义选择排序函数void selectsort(int a[],int n) 或者冒泡排序函数viod bubblesort(int a[],int n)对数组a中的元素进行排序,主函数中输入数组元素和元素个数,调用排序函数对数组元素升序排序后输出结果。分析
时间: 2024-02-05 15:12:33 浏览: 93
选择排序的实现代码如下:
```c++
void selectsort(int a[], int n) {
for (int i = 0; i < n - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < n; j++) {
if (a[j] < a[minIndex]) {
minIndex = j;
}
}
if (minIndex != i) {
int temp = a[i];
a[i] = a[minIndex];
a[minIndex] = temp;
}
}
}
```
冒泡排序的实现代码如下:
```c++
void bubblesort(int a[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - 1 - i; j++) {
if (a[j] > a[j + 1]) {
int temp = a[j];
a[j] = a[j + 1];
a[j + 1] = temp;
}
}
}
}
```
在主函数中,可以按以下方式调用排序函数:
```c++
int main() {
int a[] = {3, 1, 4, 1, 5, 9, 2, 6, 5, 3};
int n = sizeof(a) / sizeof(a[0]);
selectsort(a, n); // 或者bubblesort(a, n);
for (int i = 0; i < n; i++) {
cout << a[i] << " ";
}
cout << endl;
return 0;
}
```
这里以选择排序为例,输出结果为:
```
1 1 2 3 3 4 5 5 6 9
```
阅读全文
相关推荐
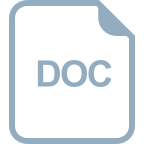
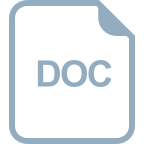
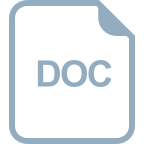













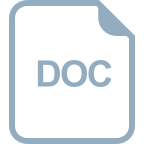
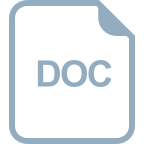
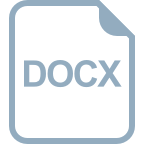