Android 自动识别物体
时间: 2023-11-21 15:57:41 浏览: 71
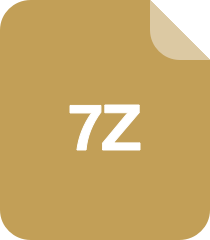
Android 手机自动识别

Android自动识别物体可以通过TensorFlow Lite实现。TensorFlow Lite是TensorFlow的轻量级版本,可以在移动设备上运行。以下是实现Android自动识别物体的步骤:
1.在Android Studio中创建一个新项目,并将TensorFlow Lite库添加到项目中。
2.下载训练好的模型,并将其添加到Android项目中。
3.在应用程序中添加相机功能,以便用户可以拍摄照片。
4.使用TensorFlow Lite模型对拍摄的照片进行分类检测。
5.将分类结果显示在应用程序中。
以下是一个简单的示例代码,用于在Android应用程序中使用TensorFlow Lite模型对拍摄的照片进行分类检测:
```java
// 加载模型
private MappedByteBuffer loadModelFile() throws IOException {
AssetFileDescriptor fileDescriptor = this.getAssets().openFd("model.tflite");
FileInputStream inputStream = new FileInputStream(fileDescriptor.getFileDescriptor());
FileChannel fileChannel = inputStream.getChannel();
long startOffset = fileDescriptor.getStartOffset();
long declaredLength = fileDescriptor.getDeclaredLength();
return fileChannel.map(FileChannel.MapMode.READ_ONLY, startOffset, declaredLength);
}
// 对拍摄的照片进行分类检测
private void classifyImage(Bitmap bitmap) {
try {
// 加载模型
MappedByteBuffer model = loadModelFile();
// 创建TensorFlow Lite解释器
Interpreter tflite = new Interpreter(model);
// 将Bitmap转换为ByteBuffer
ByteBuffer input = convertBitmapToByteBuffer(bitmap);
// 创建输出Tensor
float[][] output = new float[1][LABELS.size()];
// 运行模型
tflite.run(input, output);
// 获取分类结果
int maxIndex = getMaxIndex(output[0]);
String result = LABELS.get(maxIndex);
// 显示分类结果
textView.setText(result);
// 释放TensorFlow Lite解释器
tflite.close();
} catch (IOException e) {
e.printStackTrace();
}
}
// 将Bitmap转换为ByteBuffer
private ByteBuffer convertBitmapToByteBuffer(Bitmap bitmap) {
ByteBuffer byteBuffer = ByteBuffer.allocateDirect(4 * BATCH_SIZE * INPUT_SIZE * INPUT_SIZE * PIXEL_SIZE);
byteBuffer.order(ByteOrder.nativeOrder());
int[] pixels = new int[INPUT_SIZE * INPUT_SIZE];
bitmap.getPixels(pixels, 0, bitmap.getWidth(), 0, 0, bitmap.getWidth(), bitmap.getHeight());
int pixel = 0;
for (int i = 0; i < INPUT_SIZE; ++i) {
for (int j = 0; j < INPUT_SIZE; ++j) {
final int val = pixels[pixel++];
byteBuffer.putFloat(((val >> 16) & 0xFF) / 255.0f);
byteBuffer.putFloat(((val >> 8) & 0xFF) / 255.0f);
byteBuffer.putFloat((val & 0xFF) / 255.0f);
}
}
return byteBuffer;
}
// 获取最大值的索引
private int getMaxIndex(float[] array) {
int maxIndex = 0;
float maxVal = array[0];
for (int i = 1; i < array.length; ++i) {
if (array[i] > maxVal) {
maxVal = array[i];
maxIndex = i;
}
}
return maxIndex;
}
```
阅读全文
相关推荐
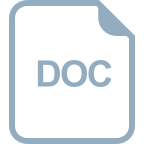
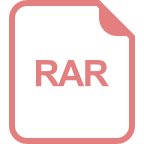
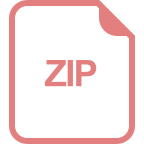
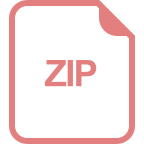
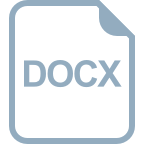
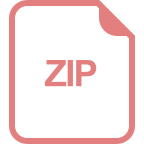
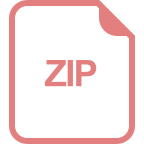
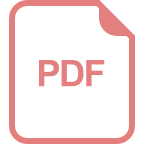
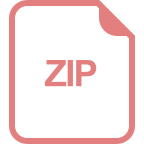
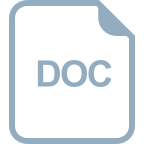
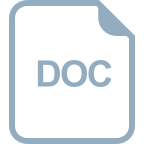
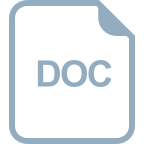
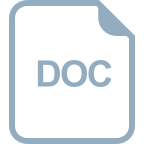
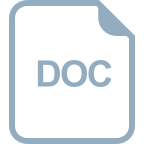
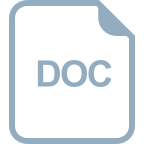
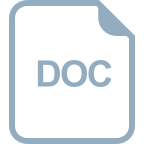