Java中保险模块赔偿代码实现
时间: 2023-08-31 20:30:55 浏览: 98
### 回答1:
保险模块的赔偿代码实现需要根据具体的业务需求进行设计和实现。下面提供一个简单的示例:
```
public class InsuranceClaim {
private int claimId;
private String policyNumber;
private String claimDescription;
private double claimAmount;
private boolean isApproved;
// Getters and setters
public int getClaimId() {
return claimId;
}
public void setClaimId(int claimId) {
this.claimId = claimId;
}
public String getPolicyNumber() {
return policyNumber;
}
public void setPolicyNumber(String policyNumber) {
this.policyNumber = policyNumber;
}
public String getClaimDescription() {
return claimDescription;
}
public void setClaimDescription(String claimDescription) {
this.claimDescription = claimDescription;
}
public double getClaimAmount() {
return claimAmount;
}
public void setClaimAmount(double claimAmount) {
this.claimAmount = claimAmount;
}
public boolean isApproved() {
return isApproved;
}
public void setApproved(boolean approved) {
isApproved = approved;
}
}
public class InsuranceClaimProcessor {
public void processClaim(InsuranceClaim claim) {
// 根据保单号查询保单信息
Policy policy = PolicyService.getPolicyByNumber(claim.getPolicyNumber());
// 判断保单是否有效
if (policy == null) {
throw new RuntimeException("保单不存在");
}
if (!policy.isActive()) {
throw new RuntimeException("保单已过期或已终止");
}
// 判断赔偿金额是否超过保额
if (claim.getClaimAmount() > policy.getCoverageAmount()) {
throw new RuntimeException("赔偿金额超过保额");
}
// 根据保单类型和赔偿原因计算赔偿金额
double payoutAmount = calculatePayout(policy.getType(), claim.getClaimDescription(), claim.getClaimAmount());
// 更新保单信息
policy.setTotalClaimAmount(policy.getTotalClaimAmount() + payoutAmount);
policy.setRemainingCoverageAmount(policy.getCoverageAmount() - policy.getTotalClaimAmount());
PolicyService.updatePolicy(policy);
// 更新赔偿申请状态
claim.setApproved(true);
}
private double calculatePayout(String policyType, String claimDescription, double claimAmount) {
// 根据保单类型和赔偿原因计算赔偿金额
// 这里简单地假设不同保单类型和赔偿原因对应不同的赔偿金额计算方法
return 0.8 * claimAmount;
}
}
public class Policy {
private String policyNumber;
private String policyType;
private double coverageAmount;
private boolean isActive;
private double totalClaimAmount;
private double remainingCoverageAmount;
// Getters and setters
public String getPolicyNumber() {
return policyNumber;
}
public void setPolicyNumber(String policyNumber) {
this.policyNumber = policyNumber;
}
public String getPolicyType() {
return policyType;
}
public void setPolicyType(String policyType) {
this.policyType = policyType;
}
public double getCoverageAmount() {
return coverageAmount;
}
public void setCoverageAmount(double coverageAmount) {
this.coverageAmount = coverageAmount;
}
public boolean isActive() {
return isActive;
}
public void setActive(boolean active) {
isActive = active;
}
public double getTotalClaimAmount() {
return totalClaimAmount;
}
public void setTotalClaimAmount(double totalClaimAmount) {
this.totalClaimAmount = totalClaimAmount;
}
public double getRemainingCoverageAmount() {
return remainingCoverageAmount;
}
public void setRemainingCoverageAmount(double remainingCoverageAmount) {
this.remainingCoverageAmount = remainingCoverageAmount;
}
}
public class PolicyService {
public static Policy getPolicyByNumber(String policyNumber) {
// 根据保单号查询保单信息,这里简单地返回一个默认的保单对象
Policy policy = new Policy();
policy.setPolicyNumber(policyNumber);
policy.setPolicyType("车险");
policy.setCoverageAmount(100000);
policy.setActive(true);
policy.setTotalClaimAmount(0);
policy.setRemainingCoverageAmount(100000);
return policy;
}
public static void updatePolicy(Policy policy) {
// 更新保单信息
}
}
```
以上代码仅作为示例,实际应用中需要根据具体的业务需求进行设计和实现。
### 回答2:
在Java中实现保险模块的赔偿代码,通常需要以下几个步骤:
1. 定义保险赔偿类:创建一个名为InsuranceClaim的类来表示保险赔偿操作。该类应包含处理赔偿请求的方法。
2. 定义保险合同类:创建一个名为InsuranceContract的类来表示保险合同,该类应包含需要的保险信息,例如保险合同号、被保险人、赔偿金额等。
3. 实现保险赔偿方法:在InsuranceClaim类中实现赔偿方法,通常需要输入保险合同号和赔偿金额作为参数。在该方法内部,可以首先通过保险合同号查询相关的保险合同信息,然后判断是否符合赔偿条件。如果满足条件,则进行赔偿操作,更新赔偿金额,并返回赔偿结果。
4. 异常处理:在保险赔偿方法中,应该考虑到可能出现的异常情况,例如保险合同号不存在、赔偿金额超过了保险金额等。可以通过使用try-catch语句来捕获异常,并针对不同的异常情况进行相应的处理,例如输出错误信息或者抛出自定义的异常类。
5. 测试代码:编写一段测试代码,用于验证保险赔偿功能的正确性。可以创建几个测试用的保险合同对象,并调用赔偿方法进行测试,验证返回结果是否符合预期。
实现保险模块的赔偿代码需要综合考虑保险合同信息的存储方式、赔偿条件的判断逻辑和错误处理等方面的问题。具体的实现方式可以根据实际需求和系统架构进行调整和扩展。以上给出的是一个基本的框架,可以根据具体情况进行修改和完善。
### 回答3:
在Java中实现保险模块赔偿的代码,首先需要定义一个保险赔偿类(InsuranceClaim),该类包含以下属性:被保险人姓名(insuredName)、保险类型(insuranceType)、赔偿金额(claimAmount)等。
在该类中,需要实现以下方法:
1. 构造方法:用于初始化被保险人姓名、保险类型和赔偿金额。
public InsuranceClaim(String insuredName, String insuranceType, double claimAmount) {
this.insuredName = insuredName;
this.insuranceType = insuranceType;
this.claimAmount = claimAmount;
}
2. 赔偿方法(claim):用于判断保险类型并计算具体赔偿金额。
public double claim() {
if (insuranceType.equals("汽车险")) {
return claimAmount * 0.8;
} else if (insuranceType.equals("健康险")) {
return claimAmount * 0.9;
} else {
return claimAmount;
}
}
3. 其他必要的getter和setter方法用于获取和设置属性值。
以上是Java中实现保险模块赔偿的简单示例代码。这段代码主要通过一个保险赔偿类来封装保险赔偿的相关数据和操作,根据不同的保险类型,计算出具体的赔偿金额。具体的赔偿规则可以根据实际需求进行修改和扩展。
阅读全文
相关推荐
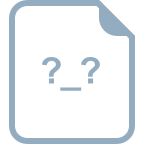
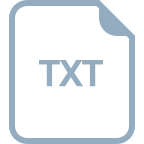
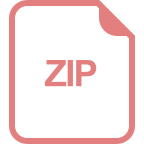















