用c# 写一个西门子ppi协议通讯库
时间: 2023-11-14 19:05:30 浏览: 231
实现西门子ppi协议通讯库可以采用以下步骤:
1. 确定通讯方式并选择相应的串口通讯库,如System.IO.Ports.SerialPort类。
2. 定义PPIMessage类,用于封装PPi协议报文。
3. 实现PPIMessage类的序列化和反序列化方法,用于将PPi协议报文转换成二进制数据和将二进制数据转换成PPi协议报文。
4. 实现PPIClient类,用于发送和接收PPi协议报文,并处理相关事件。
5. 在PPIClient类中实现连接、发送和接收方法,发送和接收方法中需要调用PPIMessage类的序列化和反序列化方法。
6. 在PPIClient类中实现事件处理方法,如接收到数据事件、连接成功事件、连接断开事件等。
7. 实现应用程序接口(API),供其他应用程序调用。
下面是一个简单的实现示例:
```csharp
using System;
using System.IO.Ports;
namespace SiemensPPI
{
// PPI消息类
public class PPIMessage
{
public byte Station { get; set; } //站号
public byte FunctionCode { get; set; } //功能码
public ushort DataLength { get; set; } //数据长度
public byte[] Data { get; set; } //数据
// 将PPI消息序列化为二进制数据
public byte[] Serialize()
{
byte[] buffer = new byte[6 + Data.Length];
buffer[0] = Station;
buffer[1] = FunctionCode;
buffer[2] = (byte)(DataLength >> 8);
buffer[3] = (byte)(DataLength & 0xFF);
Array.Copy(Data, 0, buffer, 4, Data.Length);
ushort crc = CalculateCRC(buffer, 0, buffer.Length - 2);
buffer[buffer.Length - 2] = (byte)(crc & 0xFF);
buffer[buffer.Length - 1] = (byte)(crc >> 8);
return buffer;
}
// 从二进制数据中反序列化PPI消息
public static PPIMessage Deserialize(byte[] data)
{
if (data.Length < 6)
throw new ArgumentException("Invalid message length.");
ushort crc = CalculateCRC(data, 0, data.Length - 2);
if ((data[data.Length - 2] != (byte)(crc & 0xFF)) ||
(data[data.Length - 1] != (byte)(crc >> 8)))
throw new ArgumentException("Invalid CRC.");
PPIMessage msg = new PPIMessage();
msg.Station = data[0];
msg.FunctionCode = data[1];
msg.DataLength = (ushort)((data[2] << 8) | data[3]);
msg.Data = new byte[msg.DataLength];
Array.Copy(data, 4, msg.Data, 0, msg.DataLength);
return msg;
}
// 计算CRC校验码
private static ushort CalculateCRC(byte[] data, int offset, int count)
{
ushort crc = 0xFFFF;
for (int i = offset; i < offset + count; i++)
{
crc ^= data[i];
for (int j = 0; j < 8; j++)
{
if ((crc & 0x0001) != 0)
crc = (ushort)((crc >> 1) ^ 0xA001);
else
crc >>= 1;
}
}
return crc;
}
}
// PPI客户端类
public class PPIClient
{
private SerialPort port;
private byte station;
private bool connected;
// 连接成功事件
public event EventHandler Connected;
// 连接断开事件
public event EventHandler Disconnected;
// 接收到数据事件
public event EventHandler<PPIMessage> DataReceived;
// 构造函数
public PPIClient(string portName, int baudRate, byte station)
{
port = new SerialPort(portName, baudRate);
port.DataReceived += OnDataReceived;
this.station = station;
}
// 连接到设备
public void Connect()
{
if (!port.IsOpen)
{
port.Open();
connected = true;
OnConnected();
}
}
// 断开连接
public void Disconnect()
{
if (port.IsOpen)
{
port.Close();
connected = false;
OnDisconnected();
}
}
// 发送消息
public void Send(PPIMessage message)
{
if (port.IsOpen)
{
byte[] buffer = message.Serialize();
port.Write(buffer, 0, buffer.Length);
}
}
// 接收消息
private void OnDataReceived(object sender, SerialDataReceivedEventArgs e)
{
byte[] buffer = new byte[port.BytesToRead];
port.Read(buffer, 0, buffer.Length);
PPIMessage message = PPIMessage.Deserialize(buffer);
OnDataReceived(message);
}
// 连接成功事件处理方法
private void OnConnected()
{
if (Connected != null)
Connected(this, EventArgs.Empty);
}
// 连接断开事件处理方法
private void OnDisconnected()
{
if (Disconnected != null)
Disconnected(this, EventArgs.Empty);
}
// 接收到数据事件处理方法
private void OnDataReceived(PPIMessage message)
{
if (DataReceived != null)
DataReceived(this, message);
}
}
}
```
这里只是一个简单的实现示例,如需更完整的实现请参考西门子官方文档。
阅读全文
相关推荐

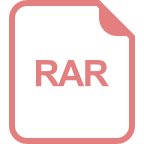














