OGR读取S57海图并转化为layer的代码
时间: 2024-03-02 18:48:01 浏览: 31
以下是使用OGR读取S-57海图并将其转换为layer的Python代码示例:
```python
from osgeo import ogr
# 打开S-57文件
s57_ds = ogr.Open('path/to/s57/file.000')
# 获取第一个图层
layer = s57_ds.GetLayerByIndex(0)
# 打印图层名称
print('Layer Name:', layer.GetName())
# 打印要素数量
print('Feature Count:', layer.GetFeatureCount())
```
这个示例中,我们首先使用`ogr.Open()`方法打开S-57文件。然后,我们使用`GetLayerByIndex()`方法获取第一个图层,您也可以通过指定图层名称来获取特定的图层。最后,我们打印图层名称和要素数量。
您可以根据需要使用其他OGR方法来进一步处理图层和要素。
相关问题
OGR读取S57海图并显示在osgearth中的代码
以下是使用 OGR 和 osgEarth 读取 S-57 海图并显示的示例代码:
```cpp
#include <osgEarth/MapNode>
#include <osgEarthAnnotation/FeatureNode>
#include <osgDB/ReadFile>
#include <ogr_api.h>
#include <ogr_s57.h>
int main(int argc, char** argv)
{
OGRRegisterAll();
// 打开 S-57 文件
OGRDataSource* ds = OGRSFDriverRegistrar::Open(argv[1], FALSE);
if (ds == NULL)
{
printf("无法打开 S-57 文件 %s\n", argv[1]);
return 1;
}
// 获取第一个图层
OGRLayer* layer = ds->GetLayer(0);
// 创建 osgEarth 的 Map 对象
osg::ref_ptr<osgEarth::Map> map = new osgEarth::Map();
// 创建一个 S57FeatureSource 对象,用于读取 S-57 数据
osg::ref_ptr<osgEarth::S57FeatureSource> s57 = new osgEarth::S57FeatureSource();
s57->setLayer(layer);
// 将 S57FeatureSource 添加到 Map 中
map->addImageLayer(s57.get());
// 加载地图文件
osg::ref_ptr<osgEarth::MapNode> mapNode = osgEarth::MapNode::create(map.get());
// 创建一个场景图结点,用于显示 S-57 数据
osg::ref_ptr<osg::Group> root = new osg::Group();
root->addChild(mapNode);
// 创建 AnnotationLayer,用于添加标注
osg::ref_ptr<osgEarth::Annotation::AnnotationLayer> annoLayer = new osgEarth::Annotation::AnnotationLayer();
mapNode->addChild(annoLayer);
// 创建 FeatureNode,用于显示要素
osg::ref_ptr<osgEarth::Annotation::FeatureNode> featureNode = new osgEarth::Annotation::FeatureNode(map.get(), s57.get());
annoLayer->addChild(featureNode);
// 运行 osgViewer
osgViewer::Viewer viewer;
viewer.setSceneData(root);
return viewer.run();
}
```
在上述代码中,首先打开 S-57 文件,然后创建一个 S57FeatureSource 对象,用于读取 S-57 数据。接着,将 S57FeatureSource 添加到 osgEarth 的 Map 对象中,并加载地图文件。最后,创建一个场景图结点,并将 FeatureNode 添加到 AnnotationLayer 中,用于显示要素。
osgearth3.2使用OGR将S57海图转化为shp并加载显示的代码
以下是使用 OGR 将 S57 海图转化为 shp 并加载显示的示例代码:
```cpp
#include <osgEarth/MapNode>
#include <osgEarth/Registry>
#include <osgEarthUtil/OGRFeatureSource>
#include <osgViewer/Viewer>
int main(int argc, char** argv)
{
// 初始化 osgEarth 应用程序
osg::ArgumentParser arguments(&argc, argv);
osg::ref_ptr<osgEarth::MapNode> mapNode = osgEarth::Util::MapNodeHelper().load(arguments, &osg::notify);
// 加载 S57 海图文件
std::string s57_file = "path/to/s57/file";
osg::ref_ptr<osgEarth::Features::FeatureSource> featureSource =
new osgEarth::Drivers::OGRFeatureOptions(s57_file, "ENC", "S57").create(mapNode->getMap());
// 将 S57 数据转换为 shp 格式
std::string shp_file = "path/to/shp/file";
osgEarth::Features::Filter filter;
filter.expression() = "FACC > 0"; // 过滤条件,只保留 FACC 大于 0 的要素
featureSource->writeFeatures(shp_file, osgEarth::Features::Format::SHP, filter);
// 创建 shp 数据源,加载并显示
osg::ref_ptr<osgEarth::Features::FeatureModelLayer> layer = new osgEarth::Features::FeatureModelLayer(
featureSource, osgEarth::Features::Filter(), osgEarth::Style::Style(), mapNode->getTerrainEngine());
mapNode->addChild(layer);
// 运行 osgViewer 应用程序
osgViewer::Viewer viewer(arguments);
viewer.setSceneData(mapNode);
return viewer.run();
}
```
需要注意的是,上述代码可能需要根据实际情况进行修改和调试。
相关推荐
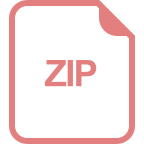












