一个84×84的ndarray,像将其变换为84×84×3的维度,用numpy的expand和repeat实现
时间: 2024-09-20 07:03:16 浏览: 46
在Python中,你可以使用NumPy库中的`numpy.expand_dims()`函数将一维数组增加一个维度,然后使用`numpy.repeat()`函数沿着新的维度复制数据,以达到改变形状的目的。假设你有一个84x84的一维数组arr,想要将其转换为84x84x3的形状,可以按照以下步骤操作:
```python
import numpy as np
# 假设 arr 是一个84x84的一维数组
arr = np.random.rand(84 * 84) # 替换为你的实际数据
# 将一维数组变成二维,新增加一个深度为1的列
expanded_arr = np.expand_dims(arr, axis=-1)
# 然后再沿新增的轴重复三次,得到84x84x3的形状
reshaped_arr = np.repeat(expanded_arr, repeats=3, axis=-1)
print("原始数组形状:", arr.shape)
print("扩展并重复后的数组形状:", reshaped_arr.shape)
```
这将会创建一个新的数组,其中每个像素都增加了三个通道值(通常是红绿蓝RGB颜色通道)。注意,你需要根据你的数据内容(是否已有颜色信息)来选择是否真的需要这么做。
相关问题
在numpy中把单通道灰度图转换成三通道
可以使用numpy的广播功能将单通道灰度图转换为三通道图像。
具体步骤如下:
1. 读取单通道灰度图像并用numpy转换为矩阵形式。
2. 使用numpy的expand_dims函数将矩阵扩展一个维度,变成三维矩阵。
3. 使用numpy的repeat函数将矩阵在新的维度上重复三次,得到三通道矩阵。
4. 将三通道矩阵转换为图像格式并保存。
示例代码如下:
```python
import numpy as np
import cv2
# 读取单通道灰度图像并用numpy转换为矩阵形式
img_gray = cv2.imread('gray.png', cv2.IMREAD_GRAYSCALE)
img_gray_mat = np.array(img_gray)
# 使用numpy的expand_dims函数将矩阵扩展一个维度,变成三维矩阵
img_gray_mat_3d = np.expand_dims(img_gray_mat, axis=2)
# 使用numpy的repeat函数将矩阵在新的维度上重复三次,得到三通道矩阵
img_color_mat = np.repeat(img_gray_mat_3d, 3, axis=2)
# 将三通道矩阵转换为图像格式并保存
img_color = cv2.cvtColor(img_color_mat, cv2.COLOR_BGR2RGB)
cv2.imwrite('color.png', img_color)
```
其中,gray.png是单通道灰度图像的文件名,color.png是转换后的三通道图像的文件名。
增加输入模型的图像集维度代码,包括batch,channel,height,width,target
好的,以下是一个示例代码,可以将一组图像数据增加维度,并将其作为模型的输入:
```python
import numpy as np
# 假设有一个存储图像数组的列表 imgs,每个数组的形状为 (height, width)
imgs = [np.ones((height, width)) for _ in range(num_images)]
targets = [np.ones((1)) for _ in range(num_images)]
# 将每个图像数组增加一个维度,并将它们拼接成一个形状为 (num_images, height, width, 1) 的三维张量
imgs_tensor = np.concatenate([np.expand_dims(img, axis=-1) for img in imgs], axis=-1)
# 将 targets 转换为一个形状为 (num_images, 1) 的二维张量
targets_tensor = np.concatenate(targets, axis=0)
targets_tensor = np.expand_dims(targets_tensor, axis=-1)
# 将 imgs_tensor 和 targets_tensor 增加一个维度,即将它们从 (num_images, height, width, 1) 和 (num_images, 1) 转换为 (batch_size, height, width, channels) 和 (batch_size, 1) 的形状
batch_size = num_images
channels = 1
imgs_tensor = np.expand_dims(imgs_tensor, axis=0)
imgs_tensor = np.repeat(imgs_tensor, batch_size, axis=0)
imgs_tensor = np.repeat(imgs_tensor, channels, axis=-1)
targets_tensor = np.expand_dims(targets_tensor, axis=0)
# 输入模型
model_input = [imgs_tensor, targets_tensor]
model_output = model.predict(model_input)
```
上述代码中,我们首先将每个图像数组增加一个维度,形成一个形状为 `(num_images, height, width, 1)` 的三维张量。然后,将这个三维张量和目标值拼接成一个元组,作为模型的输入。
接下来,我们将这个输入张量增加一个维度,即将它从 `(num_images, height, width, 1)` 转换为 `(batch_size, height, width, channels)` 的形状。这里我们假设 `batch_size= num_images`,`channels=1`。我们使用 `np.repeat` 函数来扩展张量的第一维和最后一维。
最后,我们将增加维度后的输入张量传递给模型进行预测。模型的输出结果将是一个形状为 `(batch_size, output_dim)` 的张量,其中 `output_dim` 是模型的输出维度。
阅读全文
相关推荐
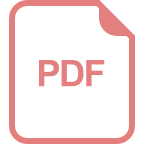
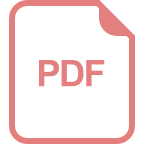
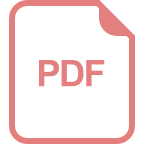
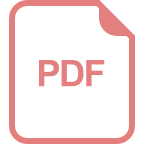
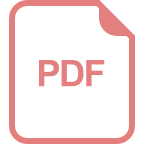



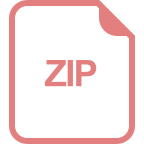
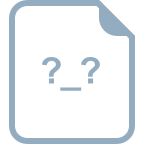
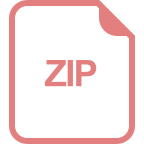
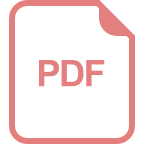
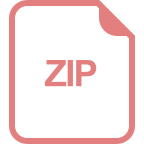
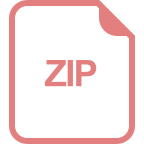
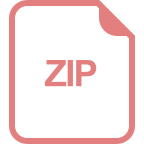