编写一个程序,输入两个字符串str1和str2,要求各串中无重复出现的字符,求两者的交集。若该交集非空,则输出结果
时间: 2024-12-20 12:20:22 浏览: 4
要编写一个C语言程序,用于找出两个字符串str1和str2中的无重复字符交集,你可以按照以下步骤进行:
1. 首先,你需要创建一个哈希集合(或称为关联数组、字典)来存储每个字符串中的唯一字符。哈希集合数据结构可以帮助你快速查找和插入元素,同时保证不会有重复。
2. 遍历第一个字符串str1,对于每个字符,检查它是否已经在哈希集合中存在。如果不存在,添加到集合中。
3. 接着遍历第二个字符串str2,同样地,对每个字符执行相同的操作。这次只添加那些不在集合中的新字符。
4. 最后,遍历哈希集合,打印出所有存在于两个字符串中的无重复字符。
这里是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdbool.h>
#include <stdlib.h>
// 哈希集合用结构体表示,使用字符作为键值对
typedef struct {
char key;
bool value;
} HashEntry;
// 创建一个哈希集合并初始化为无成员
HashEntry *createHashSet() {
return (HashEntry *)malloc(sizeof(HashEntry));
}
// 检查哈希集合中是否存在特定字符
bool isPresent(HashEntry *hashSet, char ch) {
for (int i = 0; i < sizeof(hashSet); i++) {
if (hashSet[i].key == ch && hashSet[i].value) {
return true;
}
}
return false;
}
// 插入字符到哈希集合
void insertIntoHashSet(HashEntry **hashSet, char ch) {
HashEntry *entry = createHashSet();
entry->key = ch;
entry->value = true;
// 在这里添加到实际哈希表的数据结构中,例如数组下标或链表节点
// 这里简化为数组索引,假设size=26(ASCII字母)
(*hashSet)[ch - 'a'] = *entry;
}
// 打印交集中的字符
void printIntersection(char *str1, char *str2, HashEntry *hashSet) {
for (char ch = 'a'; ch <= 'z'; ch++) {
if (hashSet[ch - 'a'].value && !isPresent(hashSet, ch)) {
printf("%c", ch);
}
}
}
int main() {
char str1[100], str2[100];
printf("Enter the first string without duplicates: ");
fgets(str1, sizeof(str1), stdin);
str1[strlen(str1) - 1] = '\0'; // remove newline character
printf("Enter the second string without duplicates: ");
fgets(str2, sizeof(str2), stdin);
str2[strlen(str2) - 1] = '\0';
HashEntry *hashSet = createHashSet();
// 将两个字符串中的无重复字符分别插入哈希集合
for (char ch : str1) {
insertIntoHashSet(&hashSet, ch);
}
for (char ch : str2) {
insertIntoHashSet(&hashSet, ch);
}
printIntersection(str1, str2, hashMap);
free(hashSet);
return 0;
}
```
阅读全文
相关推荐
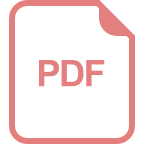
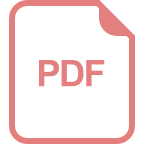
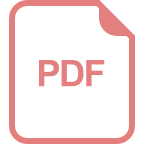















