Python字符串求交集和并集
时间: 2024-10-19 12:06:20 浏览: 37
在Python中,你可以使用集合(set)数据结构来轻松地计算两个字符串之间的交集和并集。以下是相应的操作:
1. **交集(Intersection)**:交集返回两个集合中共有的元素。对于字符串来说,它们会被转换为字符组成的集合。例如:
```python
str1 = "hello"
str2 = "world"
set1 = set(str1)
set2 = set(str2)
intersection = set1.intersection(set2)
print(intersection) # 输出:{'o', 'l'}
```
2. **并集(Union)**:并集返回两个集合的所有元素,无论是否重复。同样将字符串转换成集合再进行操作:
```python
union = set1.union(set2)
print(union) # 输出:{'w', 'r', 'd', 'h', 'e', 'l'}
```
注意,如果你需要保持原始字符串形式的交集和并集,可以先将字符串转换为列表,然后使用`list()`函数将结果转换回字符串,但这种方式不如直接使用集合高效。
相关问题
python中打开文件夹读取文件中两行字符串并获取它们的交集和并集,然后将交集和并集写入文件中
以下是一个实现上述功能的示例代码:
```python
# 打开文件夹并获取所有文件路径
import os
folder_path = '/path/to/folder'
file_paths = [os.path.join(folder_path, file) for file in os.listdir(folder_path)]
# 读取文件中的字符串
strings = []
for file_path in file_paths:
with open(file_path, 'r') as f:
lines = f.readlines()
if len(lines) >= 2:
string1 = lines[0].strip()
string2 = lines[1].strip()
strings.append((string1, string2))
# 获取交集和并集
intersection = set(strings[0][0]).intersection(strings[0][1])
union = set(strings[0][0]).union(strings[0][1])
for string1, string2 in strings[1:]:
intersection = intersection.intersection(set(string1)).intersection(set(string2))
union = union.union(set(string1)).union(set(string2))
# 将结果写入文件
with open('result.txt', 'w') as f:
f.write('Intersection: {}\n'.format(intersection))
f.write('Union: {}\n'.format(union))
```
注意,上述代码只考虑了每个文件中的前两行字符串,并假定它们都是有效的字符串。如果你的文件中可能包含无效字符串或更多的字符串,你需要修改代码以适应你的需求。
手动创建一个*.txt 文档,第一行存储 abc,第二行存储 bcd,将两行字母分别读入,以字符串形式存储;(需要考虑异常处理),2)创建一个函数,接收 1)中读取的两个字符串,返回两个字符串字符集合的交集(并集),并合成新的字符串; 3) 将 2)中合成的新字符串附加至 1)中创建的文档。
1)手动创建一个名为test.txt的文档,将以下内容复制并粘贴至文档中:
abc
bcd
2)创建一个Python函数,实现将两个字符串的字符集合合并成新字符串的功能,并返回新字符串。代码如下:
def merge_strings(str1, str2):
set1 = set(str1)
set2 = set(str2)
merged_set = set1.union(set2) # 并集
# merged_set = set1.intersection(set2) # 交集
return ''.join(sorted(list(merged_set)))
3)将2)中的函数应用于读取的两个字符串,并将结果附加至test.txt文档中。代码如下:
try:
with open('test.txt', 'r') as f:
str1 = f.readline().strip()
str2 = f.readline().strip()
merged_str = merge_strings(str1, str2)
with open('test.txt', 'a') as f:
f.write('\n' + merged_str)
except IOError:
print('文件读取错误')
except Exception as e:
print('未知错误:', e)
运行代码后,打开test.txt文档,可以看到文档最后一行已经附加了合并后的字符串。
阅读全文
相关推荐
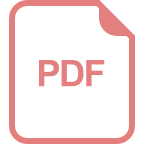
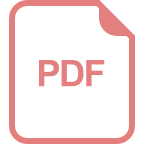
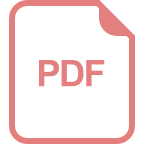



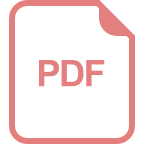
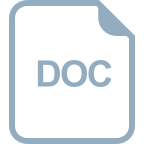
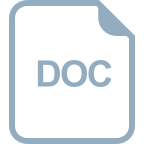
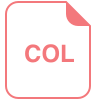
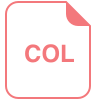




