1输入1-10的数字组成普通线性队列,2判断此时队列的队首元素,3打印出队列操作后的队首元素,4尝试用循环队列解决上述问题,用伪代码解决问题
时间: 2024-12-11 12:29:37 浏览: 11
首先,我们可以使用C++来实现一个简单的线性队列,使用数组存储队列元素,然后分别进行入队、查看队首元素、出队等操作。以下是一个基本的实现:
```cpp
#include <iostream>
class LinearQueue {
private:
int capacity;
int front, rear; // front表示队首,rear表示队尾
int* queue;
public:
LinearQueue(int size) : capacity(size), front(0), rear(0), queue(new int[capacity]) {}
~LinearQueue() { delete[] queue; }
// 入队
void enqueue(int value) {
if (rear == (front + capacity - 1) % capacity) { // 队满
std::cout << "Queue is full.\n";
return;
}
queue[rear] = value;
rear = (rear + 1) % capacity;
}
// 查看队首元素(但不删除)
bool isEmpty() const { return front == rear; }
int getFront() const {
if (isEmpty()) {
std::cout << "Queue is empty.\n";
return -1; // 返回-1代表空队列
}
return queue[front];
}
// 出队
void dequeue() {
if (isEmpty()) {
std::cout << "Queue is empty.\n";
return;
}
front = (front + 1) % capacity;
}
// 打印队首元素
void printFront() {
int frontValue = getFront();
if (frontValue != -1) {
std::cout << "The first element in the queue is: " << frontValue << "\n";
} else {
std::cout << "No elements to print.\n";
}
}
};
int main() {
LinearQueue queue(5); // 创建一个容量为5的队列
for (int i = 1; i <= 10; ++i) {
queue.enqueue(i);
queue.printFront(); // 每次入队后立即打印队首元素
}
// 循环队列实现需要使用两个指针(front和rear)和一个固定大小的数组来模拟,这里略过详细伪代码
// 基本思路是当front追上rear时,开始移动front到队列末尾继续处理。
return 0;
}
```
关于循环队列,它的实现原理是在数组的一端添加元素,在另一端移除元素,避免了动态扩容的问题。如果你希望了解如何在C++中实现循环队列,请告诉我,我会提供相应的伪代码和示例。
阅读全文
相关推荐
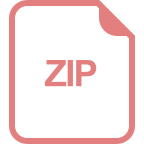
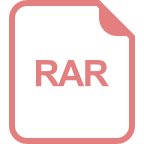
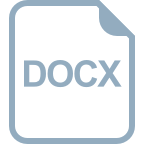















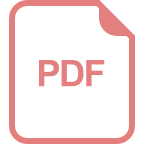