写出下面问题的C代码:要求键盘输入数据。设a[0:n-1]是一个已排好序的数组。请改写二分搜索算法,使得当搜索元素x不在数组中时,返回小于x的最大元素的位置i和大于x的最小元素位置j。当搜索元素在数组中时,i和j相同,均为x在数组中的位置。并对自己的程序进行复杂性分析。
时间: 2024-10-08 17:20:40 浏览: 17
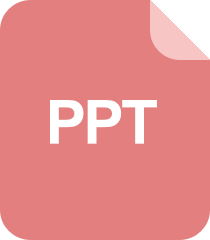
统计从键盘输入一行字符的个数-C语言学习的一个绝佳课件
```c
#include <stdio.h>
#include <limits.h>
// 函数声明
int binary_search(int arr[], int n, int x, int *less_than, int *greater_than);
int main() {
int n, i;
printf("Enter the size of the sorted array: ");
scanf("%d", &n);
// 输入排序好的整数数组
int a[n];
for (i = 0; i < n; ++i) {
printf("Element %d: ", i);
scanf("%d", &a[i]);
}
int target;
printf("Enter the element to search: ");
scanf("%d", &target);
int less_than_index, greater_than_index;
if (binary_search(a, n, target, &less_than_index, &greater_than_index)) {
printf("Element not found, less than x at index %d and greater than x at index %d.\n",
less_than_index, greater_than_index);
} else {
printf("Element found at position %d.\n", less_than_index);
}
return 0;
}
// 二分查找函数,同时更新小于和大于目标值的索引
int binary_search(int arr[], int n, int x, int *less_than, int *greater_than) {
int low = 0, high = n - 1;
while (low <= high) {
int mid = low + (high - low) / 2;
if (arr[mid] == x) {
*less_than = mid;
*greater_than = mid;
return 1; // 成功找到并返回位置
} else if (arr[mid] < x) {
*less_than = mid + 1;
low = mid + 1;
} else {
*greater_than = mid;
high = mid - 1;
}
}
return 0; // 如果没找到,返回0
}
```
阅读全文
相关推荐
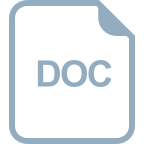
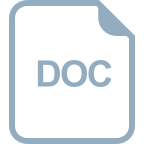
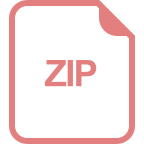
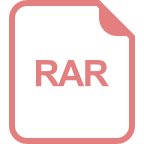
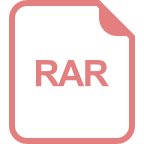
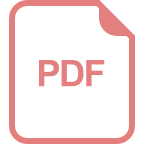
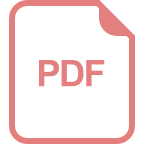
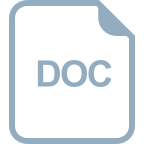
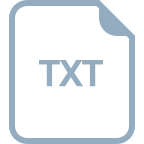
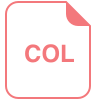
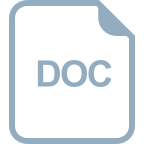
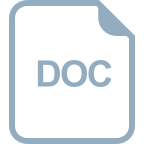
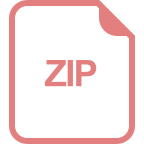
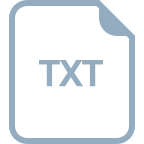
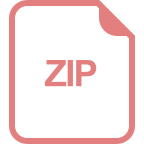
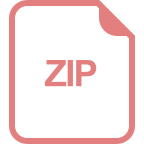
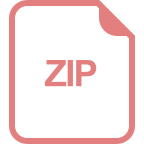