Pyqt5写一个程序,通过点击一个按钮打开一个绘制的曲线图
时间: 2024-12-25 09:30:50 浏览: 3
PyQt5是一个用于Python的应用程序开发的工具包,它结合了Qt库的GUI功能和Python的灵活性。为了创建一个简单的程序,当你点击一个按钮时显示一个绘制的曲线图,我们可以使用`QPushButton`, `QGraphicsView`以及`QPainterPath`等组件。以下是步骤和示例代码:
首先,你需要导入必要的模块:
```python
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QVBoxLayout
from PyQt5.QtGui import QPainter, QPainterPath, QColor
```
然后,定义一个窗口类并实现画图和点击事件:
```python
class CurveWindow(QWidget):
def __init__(self):
super().__init__()
self.button = QPushButton('Draw Curve', self)
self.button.clicked.connect(self.show_curve)
layout = QVBoxLayout()
layout.addWidget(self.button)
self.setLayout(layout)
def show_curve(self):
painter_path = QPainterPath()
# 这里只是一个简化的例子,你可以替换为你实际的曲线数据
points = [(0, 0), (50, 100), (100, 0), (150, 150)]
for x, y in points:
painter_path.moveTo(x, y)
painter_path.lineTo(x, y)
painter = QPainter(self)
painter.setPen(QColor(0, 0, 255)) # 设置线条颜色
painter.drawPath(painter_path)
```
最后,启动应用程序:
```python
if __name__ == '__main__':
app = QApplication([])
window = CurveWindow()
window.resize(400, 300) # 设置窗口大小
window.show()
app.exec_()
```
当你运行这个程序,会看到一个窗口,上面有一个蓝色的曲线。点击那个写着“Draw Curve”的按钮,会重新绘制曲线。
阅读全文
相关推荐
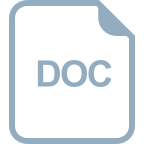
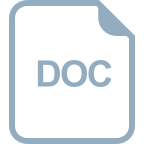
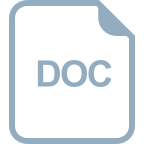
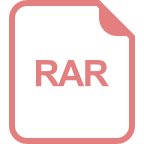
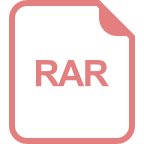
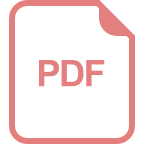
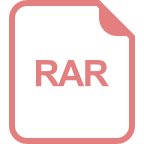
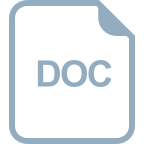
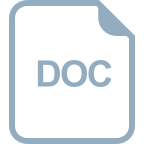
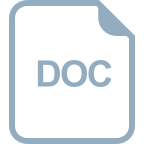
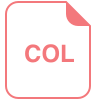






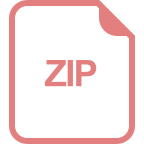
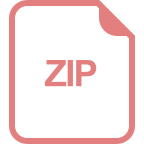