遍历pymysql结果集
时间: 2023-12-19 13:32:00 浏览: 317
遍历pymysql结果集可以使用fetchall()方法获取所有数据,然后使用for循环遍历数据。具体实现可以参考以下代码:
```python
import pymysql
# 连接数据库
link = pymysql.connect(
host='127.0.0.1', # 连接地址,连接本地默认,127.0.0.1
user='root', # 用户名
passwd='******', # 密码
port=3306, # 端口,默认为3306
db='studentdb', # 数据库名称
charset='utf8' # 字符编码
)
# 生成游标对象
cur = link.cursor()
# 写SQL语句
sql = "SELECT * FROM Student"
# 执行SQL语句
cur.execute(sql)
# 获取所有数据
data = cur.fetchall()
# 遍历数据
for i in data:
print(i)
# 关闭游标和连接
cur.close()
link.close()
```
相关问题
pymysql 字段日期
在 PyMySQL 中,日期字段可以使用 Python 中的 datetime 模块来处理。例如,如果要将日期字段插入到数据库中,可以使用以下代码:
```python
import pymysql
from datetime import datetime
# 连接数据库
conn = pymysql.connect(host='localhost', user='root', password='password', database='test')
# 获取游标
cursor = conn.cursor()
# 构造 SQL 语句
sql = "INSERT INTO table_name (date_field) VALUES (%s)"
# 定义日期变量
date = datetime.now()
# 执行 SQL 语句
cursor.execute(sql, (date,))
# 提交事务
conn.commit()
# 关闭游标和连接
cursor.close()
conn.close()
```
在查询日期字段时,可以将查询结果转换为 Python 中的 datetime 对象,例如:
```python
import pymysql
from datetime import datetime
# 连接数据库
conn = pymysql.connect(host='localhost', user='root', password='password', database='test')
# 获取游标
cursor = conn.cursor()
# 构造 SQL 语句
sql = "SELECT date_field FROM table_name"
# 执行 SQL 语句
cursor.execute(sql)
# 获取查询结果
results = cursor.fetchall()
# 遍历结果集,并将日期字段转换为 datetime 对象
for row in results:
date = datetime.strptime(row[0], '%Y-%m-%d %H:%M:%S')
print(date)
# 关闭游标和连接
cursor.close()
conn.close()
```
pymysql.cursors
pymysql.cursors模块是Python的pymysql库的一部分,它提供了一些游标对象,用于执行SQL查询并处理结果集。Pymysql库主要用于连接MySQL数据库,cursors模块的主要目的是为了增强与数据库交互的灵活性。
常见的Cursor对象有:
1. Cursor():这是最基本的游标,可以用来执行SQL语句,返回所有的查询结果。
2. DictCursor():将查询结果转换为字典,方便直接通过键访问数据。
3. SSDictCursor():同DictCursor,但它支持更高效的内存映射,对于大数据量的查询可能会更快。
4. BufferedCursor():缓冲区模式,对多次连续执行的简单查询进行优化,提高性能。
5. SSCursor():像SSDictCursor一样,同时也有缓存功能,但结果以元组形式返回。
使用这些cursor对象,你可以更方便地执行、遍历和管理数据库操作。例如:
```python
import pymysql.cursors
# 创建连接
connection = pymysql.connect(host='localhost', user='user', password='password', db='test')
try:
with connection.cursor(cursor=pymysql.cursors.DictCursor) as cursor:
# 执行查询,并以字典形式获取结果
cursor.execute("SELECT * FROM table")
results = cursor.fetchall()
for row in results:
print(row)
except:
pass
finally:
connection.close()
```
阅读全文
相关推荐
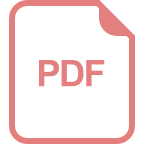
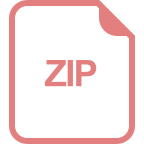
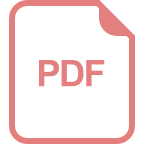
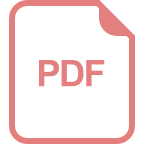
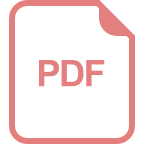
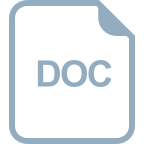
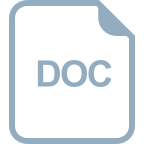
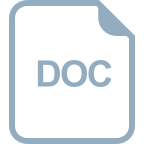
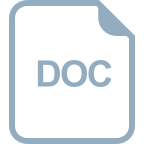
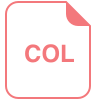





