利用Python实现IDTFT函数IDTFT (xf, nf, nT),将已知的图像逆变换生成原图像
时间: 2024-10-17 22:07:49 浏览: 37
在Python中,我们通常使用NumPy库来处理傅里叶变换(包括离散傅立叶变换,Discrete-Time Fourier Transform,简称DTFT)以及其逆运算。IDTFT(Inverse Discrete-Time Fourier Transform)用于从频域数据恢复到时域信号,类似于数字信号处理中的逆傅立叶变换。
以下是一个简单的例子,展示了如何用Python实现IDTFT:
```python
import numpy as np
def idtft(xf, nf, nT):
"""
IDTFT function using the ifft function from NumPy.
Args:
xf (numpy array): The frequency domain data.
nf (int): The number of frequencies in the input.
nT (float): Total time duration or sample length.
Returns:
np.array: The inverse transformed signal in the time domain.
"""
# Check that the input is a complex array and has correct dimensions
if not np.iscomplexobj(xf) or len(xf.shape) != 1 or xf.size % 2 == 1:
raise ValueError("Input must be a complex one-dimensional array.")
# Number of samples in the time domain (Nyquist-Shannon sampling theorem)
n = int(nf / 2 + 1)
# Perform Inverse Discrete-Time Fourier Transform (IDTFT)
t = np.linspace(0, nT, n, endpoint=False)
x_t = np.fft.ifft(xf).real
return x_t
# Example usage:
# Let's assume you have some precomputed frequency domain data `xf`
# nf and nT would depend on your specific context, e.g., if your data is sampled at 1 Hz for nT seconds
nf = ... # Your frequency count
nT = ... # Your total time
time_domain_signal = idtft(xf, nf, nT)
```
在这个例子中,`np.fft.ifft()`函数被用来计算逆变换,而`linspace()`函数创建了一个等间隔的时间序列。请注意,你需要根据实际情况提供正确的`nf`和`nT`值。
阅读全文
相关推荐
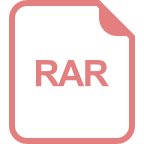
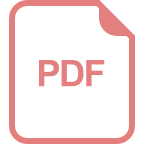
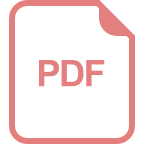
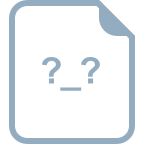
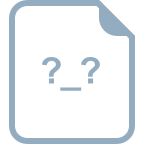
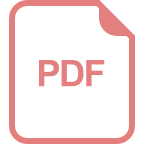
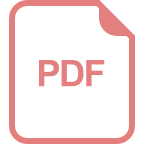
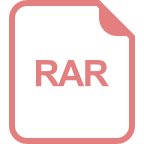
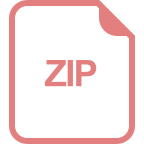
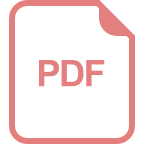
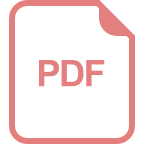
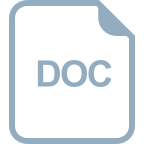
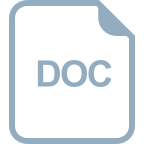
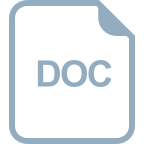
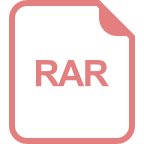
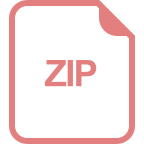