用c++实现一个qpushbutton代理
时间: 2023-11-06 21:04:14 浏览: 123
以下是一个使用C++实现QPushbutton代理的示例代码:
```
#include <QApplication>
#include <QStyledItemDelegate>
#include <QPushButton>
#include <QTableWidget>
class ButtonDelegate : public QStyledItemDelegate
{
public:
ButtonDelegate(QObject* parent = nullptr)
: QStyledItemDelegate(parent)
{}
QWidget* createEditor(QWidget* parent, const QStyleOptionViewItem& option, const QModelIndex& index) const override
{
QPushButton* button = new QPushButton(parent);
connect(button, &QPushButton::clicked, this, &ButtonDelegate::commitAndCloseEditor);
return button;
}
void setEditorData(QWidget* editor, const QModelIndex& index) const override
{
QString text = index.data().toString();
QPushButton* button = qobject_cast<QPushButton*>(editor);
if (button)
{
button->setText(text);
}
}
void commitAndCloseEditor()
{
QPushButton* button = qobject_cast<QPushButton*>(sender());
if (button)
{
emit commitData(button);
emit closeEditor(button, QAbstractItemDelegate::NoHint);
}
}
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QTableWidget table(2, 2);
ButtonDelegate delegate;
table.setItemDelegate(&delegate);
table.setItem(0, 0, new QTableWidgetItem("Click me"));
table.show();
return app.exec();
}
```
在这个示例中,我们创建了一个名为 ButtonDelegate 的代理类,继承自 QStyledItemDelegate。在 createEditor() 方法中,我们创建了一个 QPushButton 控件,并将其连接到代理的 commitAndCloseEditor() 方法。在 setEditorData() 方法中,我们从模型中获取单元格的文本,并在 QPushButton 控件上设置它。最后,在 commitAndCloseEditor() 方法中,我们发送了 commitData() 和 closeEditor() 信号,以通知模型和视图编辑已完成。
在 main() 函数中,我们创建了一个 QTableWidget 控件,将 ButtonDelegate 设置为其代理,并向单元格中添加了一个按钮。运行该应用程序后,您将看到一个表格,其中包含一个按钮单元格。单击按钮,代理将触发编辑完成的信号,并将其关闭。
阅读全文
相关推荐

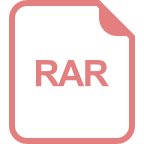











