1. 如何在Python中正确导入并使用Vector3?
时间: 2024-09-27 07:16:49 浏览: 72
在Python中,如果你想要使用像`Vector3`这样的向量类,通常它来自于游戏开发库如Pygame、Pyglet或者是三维数学库比如`numpy`或`pyquaternion`。这里以`numpy`为例,因为它是最常用的科学计算库,包含了向量数据结构:
1. 首先,你需要安装`numpy`库,如果还没有安装,可以使用pip来安装:
```
pip install numpy
```
2. 导入`numpy`中的`vectorize`函数:
```python
import numpy as np
```
3. 然后你可以创建一个`Vector3`类(虽然`numpy`本身没有这个名称,但我们假设有一个自定义的`Vector3`类):
```python
class Vector3:
def __init__(self, x=0, y=0, z=0):
self.x = x
self.y = y
self.z = z
# 定义基本操作,例如加法、减法等
def __add__(self, other):
return Vector3(self.x + other.x, self.y + other.y, self.z + other.z)
# 类似地,添加其他必要的方法
```
4. 使用`Vector3`类实例化并向其添加元素:
```python
v1 = Vector3(1, 2, 3)
v2 = Vector3(4, 5, 6)
result = v1 + v2
print(result) # 输出:Vector3(5, 7, 9)
```
注意,上述代码中的`Vector3`并不是`numpy`库的标准类,实际使用时,你可能需要查看所选库的具体文档。
阅读全文
相关推荐
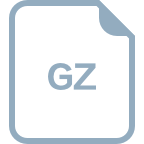

















