写一段c++内存顺序的测试代码
时间: 2024-01-18 16:04:09 浏览: 80
当涉及到内存顺序时,最常见的测试代码是使用原子操作和多线程来模拟并发访问共享资源的情况。下面是一个简单的示例代码,展示了使用C++中的atomic和thread库来测试内存顺序的效果:
```cpp
#include <iostream>
#include <atomic>
#include <thread>
#include <vector>
std::atomic<int> counter(0); // 共享计数器
void increment()
{
for (int i = 0; i < 1000; ++i)
{
counter.fetch_add(1, std::memory_order_relaxed); // relaxed内存顺序
}
}
int main()
{
std::vector<std::thread> threads;
for (int i = 0; i < 10; ++i)
{
threads.emplace_back(increment);
}
for (auto& thread : threads)
{
thread.join(); // 等待所有线程执行完成
}
std::cout << "Counter value: " << counter << std::endl;
return 0;
}
```
在上述代码中,我们使用std::atomic<int>类型的counter作为共享资源,并在多个线程中对其进行递增操作。每个线程通过调用counter.fetch_add()函数来递增计数器的值,使用std::memory_order_relaxed指定了放松顺序的内存顺序模型。
运行代码后,会创建10个线程并发执行递增操作,最终输出计数器的值。由于使用了放松顺序的内存模型,线程之间的操作可能会被重排序,因此计数器的最终值可能不是精确的10000。如果想要更严格的内存顺序保证,可以修改为其他内存顺序模型,如std::memory_order_seq_cst。
需要注意的是,代码中使用了C++11引入的原子操作和内存顺序模型,确保了对共享资源的并发访问是线程安全的。在实际开发中,需要根据具体需求选择合适的内存顺序模型来保证数据的正确性和一致性。
阅读全文
相关推荐
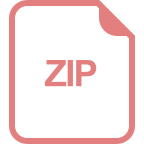
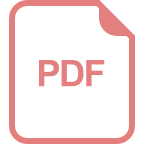
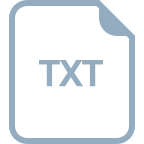









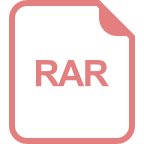
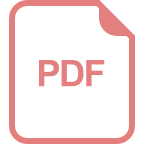
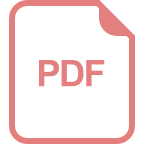
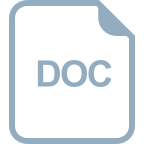
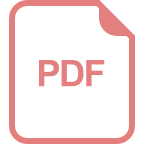
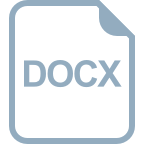