python 字典合并方法
时间: 2023-08-13 12:36:54 浏览: 65
在Python中,有多种方法可以合并字典。以下是一些常见的方法:
1. 使用`update()`方法合并字典:
```python
dict1 = {'a': 1, 'b': 2}
dict2 = {'c': 3, 'd': 4}
dict1.update(dict2)
print(dict1) # {'a': 1, 'b': 2, 'c': 3, 'd': 4}
```
2. 使用`**`运算符合并字典:
```python
dict1 = {'a': 1, 'b': 2}
dict2 = {'c': 3, 'd': 4}
merged_dict = {**dict1, **dict2}
print(merged_dict) # {'a': 1, 'b': 2, 'c': 3, 'd': 4}
```
3. 使用`dict()`和`chain()`函数合并字典:
```python
from itertools import chain
dict1 = {'a': 1, 'b': 2}
dict2 = {'c': 3, 'd': 4}
merged_dict = dict(chain(dict1.items(), dict2.items()))
print(merged_dict) # {'a': 1, 'b': 2, 'c': 3, 'd': 4}
```
4. 使用`collections`模块中的`ChainMap()`函数合并字典:
```python
from collections import ChainMap
dict1 = {'a': 1, 'b': 2}
dict2 = {'c': 3, 'd': 4}
merged_dict = dict(ChainMap(dict1, dict2))
print(merged_dict) # {'a': 1, 'b': 2, 'c': 3, 'd': 4}
```
以上是一些常见的合并字典的方法,可以根据具体的需求选择不同的方法。
相关推荐
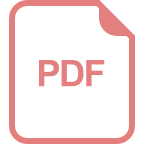
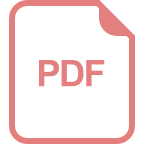
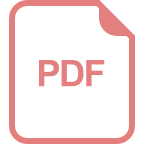














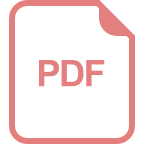