C# 给予字典中一个属性的默认值
时间: 2024-03-06 07:51:58 浏览: 189
在 C# 中,可以使用字典(Dictionary)的 TryGetValue 方法来获取一个属性的值,如果该属性不存在,则返回一个默认值。例如:
```csharp
Dictionary<string, int> myDict = new Dictionary<string, int>();
myDict.Add("a", 1);
myDict.Add("b", 2);
int defaultValue = 0;
int cValue;
if (!myDict.TryGetValue("c", out cValue))
{
cValue = defaultValue;
}
Console.WriteLine(cValue); // 输出 0,因为 'c' 属性不存在于 myDict 中
```
在上面的例子中,cValue 的值为 0,因为 'c' 属性不存在于 myDict 中,所以使用 TryGetValue 方法返回了默认值 defaultValue。
相关问题
C#中实例化一个字典并赋值
在C#中,你可以使用`Dictionary<TKey, TValue>`类来创建一个字典并实例化它。以下是如何实例化并给字典赋值的一个简单示例:
```csharp
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
// 创建一个字符串到整数的字典实例
Dictionary<string, int> studentScores = new Dictionary<string, int>();
// 实例化并添加元素
studentScores.Add("Alice", 90);
studentScores.Add("Bob", 85);
studentScores.Add("Charlie", 95);
// 访问和打印字典中的值
Console.WriteLine($"Alice's score is {studentScores["Alice"]}");
Console.WriteLine($"Bob's score is {studentScores["Bob"]}");
Console.WriteLine($"Charlie's score is {studentScores["Charlie"]}");
// 注意:如果尝试访问不存在的键,将会抛出异常
// Console.WriteLine(studentScores["David"]); // 这会引发KeyNotFoundException
// 或者可以使用TryGetValue方法安全地获取值
int score;
if (studentScores.TryGetValue("David", out score))
{
Console.WriteLine($"David's score is {score}");
}
else
{
Console.WriteLine("David is not in the dictionary.");
}
// 关闭控制台窗口
Console.ReadLine();
}
}
```
在这个例子中,我们首先定义了一个`studentScores`字典,然后添加了一些学生及其分数。可以通过键(如"Alice")来查找对应的值(分数),如果键不存在则会返回默认值或引发异常。
C# 字典中的trygetValue()
在 C# 中,`Dictionary<TKey, TValue>` 类并没有直接提供名为 `tryGetValue()` 的方法。然而,如果你是指 `Dictionary<TKey, TValue>` 的 `TryGetValue(TKey key, out TValue value)` 这个成员方法,它是一个查找键对应的值并返回结果的非阻塞操作。
这个方法的作用是在给定的键存在的情况下,尝试获取该键对应的值,并将值赋值给提供的 `out` 参数 `value`。如果键不存在,`value` 将保持其初始默认值(对于引用类型的 `TValue` 通常是 null,对于值类型则是默认构造的实例),并且方法会返回 `true`。如果键不存在,`value` 就不会被更改,方法返回 `false`。
使用 `TryGetValue()` 的示例代码如下:
```csharp
Dictionary<string, int> dict = new Dictionary<string, int>();
if (dict.TryGetValue("key", out int value))
{
// 如果 key 存在,执行这里
Console.WriteLine($"Value is {value}");
}
else
{
// key 不存在,执行这里
Console.WriteLine("Key not found");
}
```
阅读全文
相关推荐
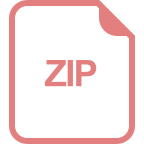
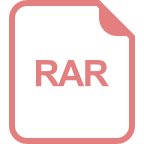
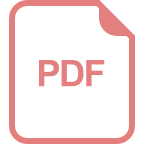
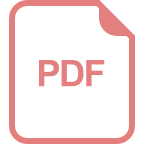
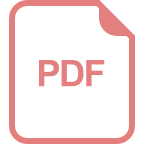
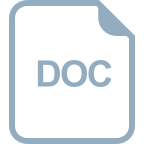
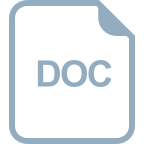
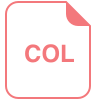
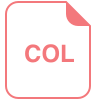
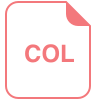
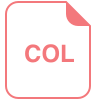




