用c语言生成完整代码实现一个简单的学生信息管理系统,该系统的功能有: 利用单链表建立学生基本信息表; 浏览每个学生的信息; 根据学号查询某个学生的基本信息; 添加学生信息到单链表中; 删除一个学生的信息。 整合在一起
时间: 2024-09-18 09:07:41 浏览: 39
以下是一个简单的C语言代码片段,实现了基本的学生信息管理系统的功能,使用了单链表结构存储学生的数据:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Student {
int id;
char name[50];
char major[50];
struct Student* next; // 指向下一个学生
} Student;
// 初始化单链表
Student* create_list() {
return NULL;
}
// 添加学生信息
void add_student(Student** head, int id, char name[], char major[]) {
Student* new_student = (Student*)malloc(sizeof(Student));
if (new_student) {
new_student->id = id;
strcpy(new_student->name, name);
strcpy(new_student->major, major);
new_student->next = *head;
*head = new_student;
} else {
printf("Memory allocation failed!\n");
}
}
// 删除学生信息
void delete_student(Student** head, int id) {
Student* current = *head;
while (current != NULL) {
if (current->id == id) {
Student* temp = current;
current = current->next;
free(temp);
break;
}
current = current->next;
}
}
// 查询学生信息
void search_student(const Student* head, int id) {
Student* current = head;
while (current != NULL) {
if (current->id == id) {
printf("ID: %d, Name: %s, Major: %s\n", current->id, current->name, current->major);
break;
}
current = current->next;
}
if (!current) {
printf("Student not found.\n");
}
}
// 浏览所有学生信息
void view_students(const Student* head) {
Student* current = head;
while (current != NULL) {
printf("ID: %d, Name: %s, Major: %s\n", current->id, current->name, current->major);
current = current->next;
}
}
int main() {
Student* student_head = create_list();
// 添加一些学生信息
add_student(&student_head, 1001, "张三", "计算机科学");
add_student(&student_head, 1002, "李四", "软件工程");
// 浏览所有学生信息
view_students(student_head);
// 根据学号查询学生
search_student(student_head, 1001);
// 删除一个学生
delete_student(&student_head, 1002);
// 再次查看学生列表
view_students(student_head);
return 0;
}
```
请注意,这个例子是基础版本,实际应用中可能需要考虑更多的错误处理和用户交互。
阅读全文
相关推荐
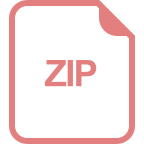
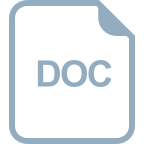

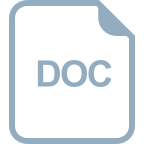
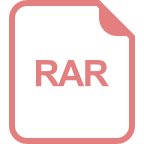
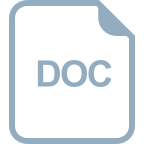
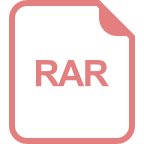
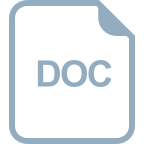
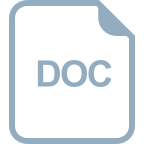
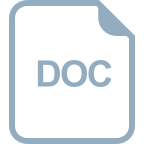
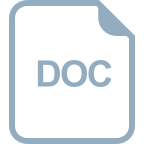

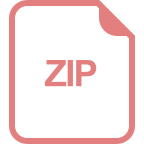
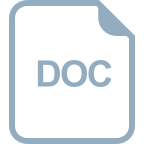
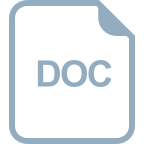
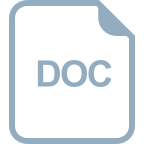
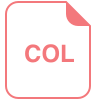
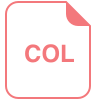